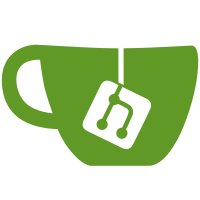
Removed outdated and unused code snippets from testfunctions.ipynb to streamline the notebook. This improves readability and reduces clutter, ensuring the file contains only relevant and functional code samples.
153 lines
4.2 KiB
Python
153 lines
4.2 KiB
Python
from fastapi.testclient import TestClient
|
|
from backend.main import app
|
|
|
|
client = TestClient(app)
|
|
|
|
|
|
def authenticate():
|
|
# Simulate login to obtain token
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "testuser", "password": "testpass"}
|
|
)
|
|
assert response.status_code == 200
|
|
return response.json()["access_token"]
|
|
|
|
|
|
def test_create_contact_success():
|
|
# Authenticate and attach token to headers
|
|
token = authenticate()
|
|
headers = {"Authorization": f"Bearer {token}"}
|
|
|
|
response = client.post(
|
|
"/protected/contacts",
|
|
headers=headers,
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
)
|
|
assert response.status_code == 201
|
|
json_response = response.json()
|
|
assert json_response["firstname"] == "John"
|
|
assert json_response["lastname"] == "Rambo"
|
|
assert json_response["email"] == "john.rambo@example.com"
|
|
|
|
|
|
def test_create_contact_unauthorized():
|
|
# Omit Authorization header to simulate unauthorized access
|
|
response = client.post(
|
|
"/protected/contacts",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
)
|
|
assert response.status_code == 401
|
|
assert response.json()["detail"] == "Not authenticated"
|
|
|
|
|
|
def test_create_contact_already_exists():
|
|
# Ensure that the route fails gracefully if contact exists
|
|
client.post(
|
|
"/contact",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
)
|
|
response = client.post(
|
|
"/contact",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
)
|
|
assert response.status_code == 400
|
|
assert (
|
|
response.json()["detail"]
|
|
== "This contact already exists in the provided pgroup."
|
|
)
|
|
|
|
|
|
def test_get_active_contacts():
|
|
# Seed with some test data
|
|
client.post(
|
|
"/contact",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
)
|
|
|
|
response = client.get("/contacts", params={"active_pgroup": "p20001"})
|
|
assert response.status_code == 200
|
|
json_response = response.json()
|
|
assert isinstance(json_response, list)
|
|
assert json_response[0]["firstname"] == "John"
|
|
assert json_response[0]["lastname"] == "Rambo"
|
|
|
|
|
|
def test_get_contacts_invalid_pgroup():
|
|
response = client.get("/contacts", params={"active_pgroup": "invalid_pgroup"})
|
|
assert response.status_code == 400
|
|
assert response.json()["detail"] == "Invalid pgroup provided."
|
|
|
|
|
|
def test_update_contact_success():
|
|
# Create a contact first
|
|
contact = client.post(
|
|
"/contact",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
).json()
|
|
|
|
response = client.put(
|
|
f"/contacts/{contact['id']}",
|
|
json={
|
|
"firstname": "Johnny",
|
|
"lastname": "Ramboski",
|
|
"pgroups": "p20001",
|
|
},
|
|
)
|
|
assert response.status_code == 200
|
|
json_response = response.json()
|
|
assert json_response["firstname"] == "Johnny"
|
|
assert json_response["lastname"] == "Ramboski"
|
|
|
|
|
|
def test_delete_contact():
|
|
# Create a contact first
|
|
contact = client.post(
|
|
"/contact",
|
|
json={
|
|
"pgroups": "p20001",
|
|
"firstname": "John",
|
|
"lastname": "Rambo",
|
|
"email": "john.rambo@example.com",
|
|
"phone_number": "+000000000",
|
|
},
|
|
).json()
|
|
|
|
response = client.delete(f"/contacts/{contact['id']}")
|
|
assert response.status_code == 204
|