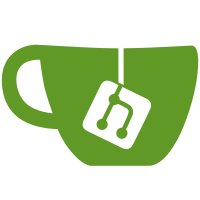
Simplify and streamline OpenAPI client generation and backend startup logic. Improved error handling, environment configuration, and self-signed SSL certificate management. Added support for generating OpenAPI schema via command-line argument.
58 lines
1.6 KiB
Bash
Executable File
58 lines
1.6 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
# Extract values from pyproject.toml
|
|
PYPROJECT_FILE="$(dirname "$0")/pyproject.toml"
|
|
|
|
NAME=$(awk -F'= ' '/^name/ { print $2 }' "$PYPROJECT_FILE" | tr -d '"')
|
|
VERSION=$(awk -F'= ' '/^version/ { print $2 }' "$PYPROJECT_FILE" | tr -d '"')
|
|
|
|
if [[ -z "$NAME" || -z "$VERSION" ]]; then
|
|
echo "Error: Unable to extract name or version from pyproject.toml."
|
|
exit 1
|
|
fi
|
|
|
|
echo "Using project name: $NAME"
|
|
echo "Using version: $VERSION"
|
|
|
|
# Navigate to backend directory
|
|
cd "$(dirname "$0")/backend" || exit
|
|
|
|
# Generate OpenAPI JSON file
|
|
echo "Generating OpenAPI JSON..."
|
|
python3 -m main generate-openapi
|
|
if [[ ! -f openapi.json ]]; then
|
|
echo "Error: Failed to generate openapi.json!"
|
|
exit 1
|
|
fi
|
|
|
|
# Download OpenAPI Generator CLI
|
|
OPENAPI_VERSION="7.8.0"
|
|
if [[ ! -f openapi-generator-cli.jar ]]; then
|
|
wget "https://repo1.maven.org/maven2/org/openapitools/openapi-generator-cli/${OPENAPI_VERSION}/openapi-generator-cli-${OPENAPI_VERSION}.jar" -O openapi-generator-cli.jar
|
|
fi
|
|
|
|
# Generate client
|
|
java -jar openapi-generator-cli.jar generate \
|
|
-i openapi.json \
|
|
-o python-client/ \
|
|
-g python \
|
|
--additional-properties=packageName="${NAME}_client",projectName="${NAME}",packageVersion="${VERSION}"
|
|
|
|
if [[ ! -d python-client ]]; then
|
|
echo "Error: Failed to generate Python client."
|
|
exit 1
|
|
fi
|
|
|
|
# Build the package
|
|
cd python-client || exit
|
|
python3 -m venv .venv
|
|
source .venv/bin/activate
|
|
pip install -U pip build
|
|
python3 -m build
|
|
|
|
if [[ ! -d dist || -z "$(ls -A dist)" ]]; then
|
|
echo "Error: Failed to build Python package."
|
|
exit 1
|
|
fi
|
|
|
|
echo "Python package built successfully in 'dist/'." |