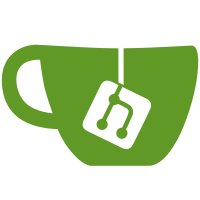
Replaced usage of "ContactPerson" with "Contact" for consistency across the codebase. Updated related component props, state variables, API calls, and database queries to align with the new model. Also enhanced backend functionality with stricter validations and added support for handling active pgroups in contact management.
40 lines
1.1 KiB
Python
40 lines
1.1 KiB
Python
# tests/test_auth.py
|
|
|
|
from fastapi.testclient import TestClient
|
|
from backend.main import app
|
|
|
|
client = TestClient(app)
|
|
|
|
|
|
def test_login_success():
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "testuser", "password": "testpass"}
|
|
)
|
|
assert response.status_code == 200
|
|
assert "access_token" in response.json()
|
|
|
|
|
|
def test_login_failure():
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "wrong", "password": "wrongpass"}
|
|
)
|
|
assert response.status_code == 401
|
|
assert response.json() == {"detail": "Incorrect username or password"}
|
|
|
|
|
|
def test_protected_route():
|
|
# Step 1: Login
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "testuser", "password": "testpass"}
|
|
)
|
|
token = response.json()["access_token"]
|
|
|
|
# Step 2: Access protected route
|
|
headers = {"Authorization": f"Bearer {token}"}
|
|
response = client.get("/auth/protected-route", headers=headers)
|
|
assert response.status_code == 200
|
|
assert response.json() == {
|
|
"username": "testuser",
|
|
"pgroups": [20000, 20001, 20002, 20003],
|
|
}
|