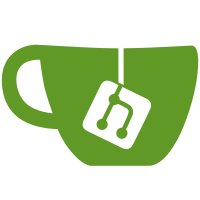
Introduced a new `local_contact_router` to handle creation of local contacts. The endpoint enforces role-based access control and ensures no duplication of email addresses. Updated the router exports for consistency and cleaned up a large test file to improve readability.
57 lines
1.8 KiB
Python
57 lines
1.8 KiB
Python
from fastapi import APIRouter, HTTPException, status, Depends
|
|
from sqlalchemy.orm import Session
|
|
|
|
from app.models import LocalContact as LocalContactModel
|
|
from app.schemas import LocalContactCreate as LocalContactSchema, loginData
|
|
from app.dependencies import get_db
|
|
from app.routers.auth import get_current_user
|
|
|
|
local_contact_router = APIRouter()
|
|
|
|
|
|
@local_contact_router.post(
|
|
"/",
|
|
response_model=LocalContactSchema,
|
|
status_code=status.HTTP_201_CREATED,
|
|
)
|
|
async def create_local_contact(
|
|
local_contact: LocalContactSchema,
|
|
db: Session = Depends(get_db),
|
|
current_user: loginData = Depends(get_current_user),
|
|
):
|
|
"""
|
|
Create a new local contact. Only selected users can create a local contact.
|
|
"""
|
|
# Access control: Only allow users with specific roles (e.g., "admin" or
|
|
# "contact_manager")
|
|
if current_user.role not in ["admin", "contact_manager"]:
|
|
raise HTTPException(
|
|
status_code=status.HTTP_403_FORBIDDEN,
|
|
detail="You do not have permission to create a local contact.",
|
|
)
|
|
|
|
# Check if a local contact with the same email already exists
|
|
if (
|
|
db.query(LocalContactModel)
|
|
.filter(LocalContactModel.email == local_contact.email)
|
|
.first()
|
|
):
|
|
raise HTTPException(
|
|
status_code=status.HTTP_400_BAD_REQUEST,
|
|
detail="A local contact with this email already exists.",
|
|
)
|
|
|
|
# Create a new LocalContact
|
|
db_local_contact = LocalContactModel(
|
|
firstname=local_contact.firstname,
|
|
lastname=local_contact.lastname,
|
|
phone_number=local_contact.phone_number,
|
|
email=local_contact.email,
|
|
status=local_contact.status or "active",
|
|
)
|
|
db.add(db_local_contact)
|
|
db.commit()
|
|
db.refresh(db_local_contact)
|
|
|
|
return db_local_contact
|