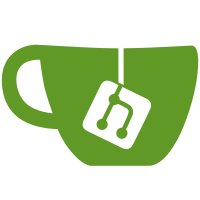
Enhance app with active pgroup handling and token updates Added active pgroup state management across the app for user-specific settings. Improved token handling with decoding, saving user data, and setting OpenAPI authorization. Updated components, API calls, and forms to support dynamic pgroup selection and user-specific features.
199 lines
8.9 KiB
TypeScript
199 lines
8.9 KiB
TypeScript
import React, { useState } from 'react';
|
|
import { useNavigate, useLocation } from 'react-router-dom';
|
|
import AppBar from '@mui/material/AppBar';
|
|
import Box from '@mui/material/Box';
|
|
import Toolbar from '@mui/material/Toolbar';
|
|
import IconButton from '@mui/material/IconButton';
|
|
import Typography from '@mui/material/Typography';
|
|
import Menu from '@mui/material/Menu';
|
|
import MenuItem from '@mui/material/MenuItem';
|
|
import Container from '@mui/material/Container';
|
|
import Avatar from '@mui/material/Avatar';
|
|
import Tooltip from '@mui/material/Tooltip';
|
|
import { Button, Select, FormControl, InputLabel, } from '@mui/material';
|
|
import { Link } from 'react-router-dom';
|
|
import logo from '../assets/icons/psi_01_sn.svg';
|
|
import '../App.css';
|
|
import { clearToken } from '../utils/auth';
|
|
|
|
|
|
interface ResponsiveAppBarProps {
|
|
activePgroup: string;
|
|
onOpenAddressManager: () => void;
|
|
onOpenContactsManager: () => void;
|
|
pgroups: string[]; // Pass the pgroups from the server
|
|
currentPgroup: string; // Currently selected pgroup
|
|
onPgroupChange: (pgroup: string) => void; // Callback when selected pgroup changes
|
|
}
|
|
|
|
const ResponsiveAppBar: React.FC<ResponsiveAppBarProps> = ({
|
|
activePgroup,
|
|
onOpenAddressManager,
|
|
onOpenContactsManager,
|
|
pgroups,
|
|
currentPgroup,
|
|
onPgroupChange }) => {
|
|
const navigate = useNavigate();
|
|
const location = useLocation();
|
|
const [anchorElNav, setAnchorElNav] = useState<null | HTMLElement>(null);
|
|
const [anchorElUser, setAnchorElUser] = useState<null | HTMLElement>(null);
|
|
const [selectedPgroup, setSelectedPgroup] = useState(currentPgroup);
|
|
console.log('Active Pgroup:', activePgroup);
|
|
const handlePgroupChange = (event: React.ChangeEvent<{ value: unknown }>) => {
|
|
const newPgroup = event.target.value as string;
|
|
setSelectedPgroup(newPgroup);
|
|
onPgroupChange(newPgroup); // Inform parent about the change
|
|
};
|
|
|
|
const pages = [
|
|
{ name: 'Home', path: '/' },
|
|
{ name: 'Shipments', path: '/shipments' },
|
|
{ name: 'Planning', path: '/planning' },
|
|
{ name: 'Results', path: '/results' }
|
|
];
|
|
|
|
const userMenuItems = [
|
|
{ name: 'My Contacts', action: 'contacts' },
|
|
{ name: 'My Addresses', action: 'addresses' },
|
|
{ name: 'DUO', path: '/duo' },
|
|
{ name: 'Logout', action: 'logout' }
|
|
];
|
|
|
|
const handleOpenNavMenu = (event: React.MouseEvent<HTMLElement>) => {
|
|
setAnchorElNav(event.currentTarget);
|
|
};
|
|
|
|
const handleCloseNavMenu = () => {
|
|
setAnchorElNav(null);
|
|
};
|
|
|
|
const handleOpenUserMenu = (event: React.MouseEvent<HTMLElement>) => {
|
|
setAnchorElUser(event.currentTarget);
|
|
};
|
|
|
|
const handleCloseUserMenu = () => {
|
|
setAnchorElUser(null);
|
|
};
|
|
|
|
const handleLogout = () => {
|
|
console.log("Performing logout...");
|
|
clearToken(); // Clear the token from localStorage and OpenAPI
|
|
navigate('/login'); // Redirect to login page
|
|
};
|
|
|
|
const handleMenuItemClick = (action: string) => {
|
|
if (action === 'contacts') {
|
|
onOpenContactsManager();
|
|
} else if (action === 'addresses') {
|
|
onOpenAddressManager();
|
|
} else if (action === 'logout') {
|
|
handleLogout();
|
|
}
|
|
handleCloseUserMenu();
|
|
};
|
|
|
|
return (
|
|
<div>
|
|
<AppBar position="static" sx={{ backgroundColor: '#324A5F' }}>
|
|
<Container maxWidth="xl">
|
|
<Toolbar disableGutters>
|
|
<Link to="/" style={{ textDecoration: 'none', display: 'flex', alignItems: 'center' }}>
|
|
<img src={logo} height="40px" alt="PSI logo" style={{ marginRight: 12 }}/>
|
|
<Typography
|
|
variant="h6"
|
|
noWrap
|
|
sx={{
|
|
display: 'flex',
|
|
fontFamily: 'Roboto, sans-serif',
|
|
fontWeight: 700,
|
|
color: 'inherit',
|
|
textDecoration: 'none',
|
|
transition: 'color 0.3s',
|
|
'&:hover': {
|
|
color: '#f0db4f',
|
|
},
|
|
}}
|
|
>
|
|
AARE v0.1
|
|
</Typography>
|
|
</Link>
|
|
|
|
<Box sx={{ flexGrow: 1, display: { xs: 'none', md: 'flex' }, justifyContent: 'center' }}>
|
|
{pages.map((page) => (
|
|
<Button
|
|
key={page.name}
|
|
component={Link}
|
|
to={page.path}
|
|
sx={{
|
|
mx: 2,
|
|
color: page.path === location.pathname ? '#f0db4f' : 'white',
|
|
fontWeight: page.path === location.pathname ? 700 : 500,
|
|
textTransform: 'none',
|
|
fontSize: '1rem',
|
|
transition: 'color 0.3s, background-color 0.3s',
|
|
'&:hover': {
|
|
color: '#f0db4f',
|
|
backgroundColor: 'rgba(255,255,255,0.1)',
|
|
},
|
|
}}
|
|
>
|
|
{page.name}
|
|
</Button>
|
|
))}
|
|
</Box>
|
|
<Box>
|
|
<FormControl variant="outlined" size="small">
|
|
<InputLabel sx={{ color: 'white' }}>pgroup</InputLabel>
|
|
<Select
|
|
value={selectedPgroup}
|
|
onChange={handlePgroupChange}
|
|
sx={{
|
|
color: 'white',
|
|
borderColor: 'white',
|
|
minWidth: 150,
|
|
'&:focus': { borderColor: '#f0db4f' },
|
|
}}
|
|
>
|
|
{pgroups && pgroups.length > 0 ? (
|
|
pgroups.map((pgroup) => (
|
|
<MenuItem key={pgroup} value={pgroup}>
|
|
{pgroup}
|
|
</MenuItem>
|
|
))
|
|
) : (
|
|
<MenuItem disabled>No pgroups available</MenuItem>
|
|
)}
|
|
</Select>
|
|
</FormControl>
|
|
</Box>
|
|
<Box sx={{ flexGrow: 0 }}>
|
|
<Tooltip title="Open settings">
|
|
<IconButton onClick={handleOpenUserMenu} sx={{ p: 0 }}>
|
|
<Avatar sx={{ bgcolor: 'yellowgreen' }} />
|
|
</IconButton>
|
|
</Tooltip>
|
|
<Menu
|
|
sx={{ mt: '45px' }}
|
|
id="menu-appbar"
|
|
anchorEl={anchorElUser}
|
|
anchorOrigin={{ vertical: 'top', horizontal: 'right' }}
|
|
keepMounted
|
|
transformOrigin={{ vertical: 'top', horizontal: 'right' }}
|
|
open={Boolean(anchorElUser)}
|
|
onClose={handleCloseUserMenu}
|
|
>
|
|
{userMenuItems.map((item) => (
|
|
<MenuItem key={item.name} onClick={() => handleMenuItemClick(item.action ?? '')}>
|
|
{item.name}
|
|
</MenuItem>
|
|
))}
|
|
</Menu>
|
|
</Box>
|
|
</Toolbar>
|
|
</Container>
|
|
</AppBar>
|
|
</div>
|
|
);
|
|
};
|
|
|
|
export default ResponsiveAppBar; |