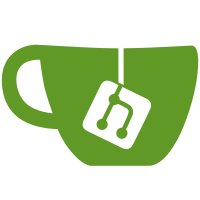
Introduced `mount_count` and `unmount_count` fields to track mounting events for samples. Updated models, schemas, and front-end components to support dynamic calculation and display of these counts. Enhanced backend queries and API responses to include the new data.
602 lines
39 KiB
Plaintext
602 lines
39 KiB
Plaintext
{
|
|
"cells": [
|
|
{
|
|
"cell_type": "code",
|
|
"id": "initial_id",
|
|
"metadata": {
|
|
"collapsed": true,
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:03:52.460891Z",
|
|
"start_time": "2025-01-17T14:03:52.454842Z"
|
|
}
|
|
},
|
|
"source": [
|
|
"import json\n",
|
|
"\n",
|
|
"from nbclient.client import timestamp\n",
|
|
"\n",
|
|
"import backend.aareDBclient as aareDBclient\n",
|
|
"from aareDBclient.rest import ApiException\n",
|
|
"from pprint import pprint\n",
|
|
"\n",
|
|
"from app.data.data import sample\n",
|
|
"\n",
|
|
"#from aareDBclient import SamplesApi, ShipmentsApi, PucksApi\n",
|
|
"#from aareDBclient.models import SampleEventCreate, SetTellPosition\n",
|
|
"#from examples.examples import api_response\n",
|
|
"\n",
|
|
"print(aareDBclient.__version__)\n",
|
|
"\n",
|
|
"configuration = aareDBclient.Configuration(\n",
|
|
" #host = \"https://mx-aare-test.psi.ch:1492\"\n",
|
|
" host = \"https://127.0.0.1:8000\"\n",
|
|
")\n",
|
|
"\n",
|
|
"print(configuration.host)\n",
|
|
"\n",
|
|
"configuration.verify_ssl = False # Disable SSL verification\n",
|
|
"#print(dir(SamplesApi))"
|
|
],
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"0.1.0a19\n",
|
|
"https://127.0.0.1:8000\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 40
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:13:16.559702Z",
|
|
"start_time": "2025-01-17T14:13:16.446021Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"## Fetch all Shipments, list corresponding dewars and pucks\n",
|
|
"\n",
|
|
"from datetime import date, datetime\n",
|
|
"from aareDBclient import ShipmentsApi\n",
|
|
"from aareDBclient.models import Shipment\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.ShipmentsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Fetch all shipments\n",
|
|
" all_shipments_response = api_instance.fetch_shipments_shipments_get()\n",
|
|
"\n",
|
|
" # Print shipment names and their associated puck names\n",
|
|
" for shipment in all_shipments_response:\n",
|
|
" print(f\"Shipment ID: {shipment.id}, Shipment Name: {shipment.shipment_name}\")\n",
|
|
" if hasattr(shipment, 'dewars') and shipment.dewars: # Ensure 'dewars' exists\n",
|
|
" for dewar in shipment.dewars:\n",
|
|
" print(f\" Dewar ID: {dewar.id}, Dewar Name: {dewar.dewar_name}, Dewar Unique ID: {dewar.unique_id} \")\n",
|
|
"\n",
|
|
" if hasattr(dewar, 'pucks') and dewar.pucks: # Ensure 'pucks' exists\n",
|
|
" for puck in dewar.pucks:\n",
|
|
" print(f\" Puck ID: {puck.id}, Puck Name: {puck.puck_name}\")\n",
|
|
" else:\n",
|
|
" print(\" No pucks found in this dewar.\")\n",
|
|
" else:\n",
|
|
" print(\" No dewars found in this shipment.\")\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling ShipmentsApi->fetch_shipments_shipments_get: {e}\")\n"
|
|
],
|
|
"id": "45cc7ab6d4589711",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Shipment ID: 2, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 1, Dewar Name: Dewar One, Dewar Unique ID: 83041c3f8844ff39 \n",
|
|
" Puck ID: 1, Puck Name: PUCK-001\n",
|
|
" Puck ID: 2, Puck Name: PUCK002\n",
|
|
" Puck ID: 3, Puck Name: PUCK003\n",
|
|
" Puck ID: 4, Puck Name: PUCK004\n",
|
|
" Puck ID: 5, Puck Name: PUCK005\n",
|
|
" Puck ID: 6, Puck Name: PUCK006\n",
|
|
" Puck ID: 7, Puck Name: PUCK007\n",
|
|
" Dewar ID: 2, Dewar Name: Dewar Two, Dewar Unique ID: 62baccecdd37c033 \n",
|
|
" Puck ID: 8, Puck Name: PK001\n",
|
|
" Puck ID: 9, Puck Name: PK002\n",
|
|
" Puck ID: 10, Puck Name: PK003\n",
|
|
" Puck ID: 11, Puck Name: PK004\n",
|
|
" Puck ID: 12, Puck Name: PK005\n",
|
|
" Puck ID: 13, Puck Name: PK006\n",
|
|
"Shipment ID: 3, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 3, Dewar Name: Dewar Three, Dewar Unique ID: None \n",
|
|
" Puck ID: 14, Puck Name: P001\n",
|
|
" Puck ID: 15, Puck Name: P002\n",
|
|
" Puck ID: 16, Puck Name: P003\n",
|
|
" Puck ID: 17, Puck Name: P004\n",
|
|
" Puck ID: 18, Puck Name: P005\n",
|
|
" Puck ID: 19, Puck Name: P006\n",
|
|
" Puck ID: 20, Puck Name: P007\n",
|
|
" Dewar ID: 4, Dewar Name: Dewar Four, Dewar Unique ID: None \n",
|
|
" Puck ID: 21, Puck Name: PC002\n",
|
|
" Puck ID: 22, Puck Name: PC003\n",
|
|
" Puck ID: 23, Puck Name: PC004\n",
|
|
" Puck ID: 24, Puck Name: PC005\n",
|
|
" Puck ID: 25, Puck Name: PC006\n",
|
|
" Puck ID: 26, Puck Name: PC007\n",
|
|
"Shipment ID: 1, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 5, Dewar Name: Dewar Five, Dewar Unique ID: 15e3dbe05e78ee83 \n",
|
|
" Puck ID: 27, Puck Name: PKK004\n",
|
|
" Puck ID: 28, Puck Name: PKK005\n",
|
|
" Puck ID: 29, Puck Name: PKK006\n",
|
|
" Puck ID: 30, Puck Name: PKK007\n",
|
|
"Shipment ID: 4, Shipment Name: testship\n",
|
|
" Dewar ID: 6, Dewar Name: Dewar_test, Dewar Unique ID: f352529444d64dd5 \n",
|
|
" Puck ID: 43, Puck Name: CPS-4093\n",
|
|
" Puck ID: 44, Puck Name: CPS-4178\n",
|
|
" Puck ID: 45, Puck Name: PSIMX-122\n",
|
|
" Puck ID: 46, Puck Name: CPS-6597\n",
|
|
" Puck ID: 47, Puck Name: PSIMX-078\n",
|
|
" Puck ID: 48, Puck Name: 1002\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 44
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:14:30.201369Z",
|
|
"start_time": "2025-01-17T14:14:30.175577Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from datetime import date\n",
|
|
"from aareDBclient import LogisticsApi\n",
|
|
"from aareDBclient.models import LogisticsEventCreate # Import required model\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.LogisticsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='incoming',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='refill',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='X06DA-Beamline',\n",
|
|
" transaction_type='beamline',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n"
|
|
],
|
|
"id": "f5de1787214a6642",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 45
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:07:51.580993Z",
|
|
"start_time": "2025-01-17T14:07:51.565128Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Get a list of pucks that are \"at the beamline\"\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create Return Address\n",
|
|
" api_response = api_instance.get_pucks_by_slot_pucks_slot_slot_identifier_get(slot_identifier='X06DA')\n",
|
|
" print(\"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get: %s\\n\" % e)"
|
|
],
|
|
"id": "bbee7c94bf14000c",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\n",
|
|
"\n",
|
|
"[PuckWithTellPosition(id=43, puck_name='CPS-4093', puck_type='unipuck', puck_location_in_dewar=1, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=44, puck_name='CPS-4178', puck_type='unipuck', puck_location_in_dewar=2, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=45, puck_name='PSIMX-122', puck_type='unipuck', puck_location_in_dewar=3, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=46, puck_name='CPS-6597', puck_type='unipuck', puck_location_in_dewar=4, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=47, puck_name='PSIMX-078', puck_type='unipuck', puck_location_in_dewar=5, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=48, puck_name='1002', puck_type='unipuck', puck_location_in_dewar=6, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None)]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 42
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:09:55.141237Z",
|
|
"start_time": "2025-01-17T14:09:55.117843Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Attribute a puck to a position in the TELL dewar\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # This part is commented but will be used to attribute a puck to a position of the TELL\n",
|
|
" # Define the puck ID and payload\n",
|
|
"\n",
|
|
" payload = [SetTellPosition(puck_name='CPS-4178', segment='A', puck_in_segment=2),SetTellPosition(puck_name='PSIMX122', segment='C', puck_in_segment=3)]\n",
|
|
" #payload = []\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Call the PUT method to update the tell_position\n",
|
|
" api_response = api_instance.set_tell_positions_pucks_set_tell_positions_put(payload)\n",
|
|
" print(\"The response of PucksApi->pucks_puck_id_tell_position_put:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->pucks_puck_id_tell_position_put: %s\\n\" % e)"
|
|
],
|
|
"id": "d52d12287dd63299",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->pucks_puck_id_tell_position_put:\n",
|
|
"\n",
|
|
"[{'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'A2',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'CPS-4178',\n",
|
|
" 'status': 'updated'},\n",
|
|
" {'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'C3',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'PSIMX-122',\n",
|
|
" 'status': 'updated'}]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 43
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-20T10:00:34.361066Z",
|
|
"start_time": "2025-01-20T10:00:34.339557Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Get puck_id puck_name sample_id sample_name of pucks in the tell\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # GET request: Fetch all pucks in the tell\n",
|
|
" try:\n",
|
|
" # Call the API method to fetch pucks\n",
|
|
" all_pucks_response = api_instance.get_pucks_with_tell_position_pucks_with_tell_position_get()\n",
|
|
"\n",
|
|
" # Debug response structure by printing it in JSON format\n",
|
|
" formatted_response = json.dumps(\n",
|
|
" [p.to_dict() for p in all_pucks_response], # Assuming the API response can be converted to dicts\n",
|
|
" indent=4 # Use indentation for readability\n",
|
|
" )\n",
|
|
" #print(\"The response of PucksApi->get_all_pucks_in_tell (formatted):\\n\")\n",
|
|
" #print(formatted_response)\n",
|
|
"\n",
|
|
" # Iterate through each puck and print information\n",
|
|
" for p in all_pucks_response:\n",
|
|
" print(f\"Puck ID: {p.id}, Puck Name: {p.puck_name}\")\n",
|
|
"\n",
|
|
" # Check if the puck has any samples\n",
|
|
" if hasattr(p, 'samples') and p.samples: # Ensure 'samples' attribute exists and is not empty\n",
|
|
" for sample in p.samples:\n",
|
|
" print(f\" Sample ID: {sample.id}, Sample Name: {sample.sample_name}\")\n",
|
|
" else:\n",
|
|
" print(\" No samples found in this puck.\")\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_all_pucks_in_tell: %s\\n\" % e)"
|
|
],
|
|
"id": "95f8c133359945d5",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Puck ID: 44, Puck Name: CPS-4178\n",
|
|
" Sample ID: 433, Sample Name: Dtpase_1\n",
|
|
" Sample ID: 434, Sample Name: Dtpase_2\n",
|
|
" Sample ID: 435, Sample Name: Dtpase_3\n",
|
|
" Sample ID: 436, Sample Name: Dtpase_4\n",
|
|
" Sample ID: 437, Sample Name: Dtpase_5\n",
|
|
" Sample ID: 438, Sample Name: Dtpase_6\n",
|
|
" Sample ID: 439, Sample Name: Dtpase_7\n",
|
|
" Sample ID: 440, Sample Name: Fckase_1\n",
|
|
" Sample ID: 441, Sample Name: Fckase_2\n",
|
|
" Sample ID: 442, Sample Name: Fckase_3\n",
|
|
" Sample ID: 443, Sample Name: Fckase_4\n",
|
|
" Sample ID: 444, Sample Name: Fckase_5\n",
|
|
" Sample ID: 445, Sample Name: Fckase_6\n",
|
|
" Sample ID: 446, Sample Name: Fckase_7\n",
|
|
" Sample ID: 447, Sample Name: Fckase_8\n",
|
|
" Sample ID: 448, Sample Name: Fckase_9\n",
|
|
"Puck ID: 45, Puck Name: PSIMX-122\n",
|
|
" Sample ID: 449, Sample Name: PopoI_1\n",
|
|
" Sample ID: 450, Sample Name: PopoI_2\n",
|
|
" Sample ID: 451, Sample Name: PopoI_3\n",
|
|
" Sample ID: 452, Sample Name: PopoI_4\n",
|
|
" Sample ID: 453, Sample Name: PopoI_5\n",
|
|
" Sample ID: 454, Sample Name: PopoI_6\n",
|
|
" Sample ID: 455, Sample Name: PopoI_7\n",
|
|
" Sample ID: 456, Sample Name: PopoI_8\n",
|
|
" Sample ID: 457, Sample Name: PopoI_9\n",
|
|
" Sample ID: 458, Sample Name: PopoI_10\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 53
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-20T11:10:20.017201Z",
|
|
"start_time": "2025-01-20T11:10:19.953940Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from aareDBclient import SampleEventCreate\n",
|
|
"\n",
|
|
"# Create an event for a sample\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Instance of the API client\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Define the payload with only `event_type`\n",
|
|
" sample_event_create = SampleEventCreate(\n",
|
|
" sample_id=433,\n",
|
|
" event_type=\"Mounted\" # Valid event type\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Debug the payload before sending\n",
|
|
" print(\"Payload being sent to API:\")\n",
|
|
" print(sample_event_create.json()) # Ensure it matches `SampleEventCreate`\n",
|
|
"\n",
|
|
" # Call the API\n",
|
|
" api_response = api_instance.create_sample_event_samples_samples_sample_id_events_post(\n",
|
|
" sample_id=433, # Ensure this matches a valid sample ID in the database\n",
|
|
" sample_event_create=sample_event_create\n",
|
|
" )\n",
|
|
"\n",
|
|
" print(\"API response:\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling post_sample_event:\")\n",
|
|
" print(f\"Status Code: {e.status}\")\n",
|
|
" if e.body:\n",
|
|
" print(f\"Error Details: {e.body}\")\n"
|
|
],
|
|
"id": "ee8abb293096334a",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Payload being sent to API:\n",
|
|
"{\"event_type\":\"Mounted\"}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
},
|
|
{
|
|
"ename": "ValidationError",
|
|
"evalue": "3 validation errors for SampleEventResponse\nsample_id\n Input should be a valid integer [type=int_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/int_type\nevent_type\n Input should be a valid string [type=string_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/string_type\ntimestamp\n Input should be a valid datetime [type=datetime_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/datetime_type",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mValidationError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[110], line 21\u001B[0m\n\u001B[1;32m 18\u001B[0m \u001B[38;5;28mprint\u001B[39m(sample_event_create\u001B[38;5;241m.\u001B[39mjson()) \u001B[38;5;66;03m# Ensure it matches `SampleEventCreate`\u001B[39;00m\n\u001B[1;32m 20\u001B[0m \u001B[38;5;66;03m# Call the API\u001B[39;00m\n\u001B[0;32m---> 21\u001B[0m api_response \u001B[38;5;241m=\u001B[39m \u001B[43mapi_instance\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mcreate_sample_event_samples_samples_sample_id_events_post\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 22\u001B[0m \u001B[43m \u001B[49m\u001B[43msample_id\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[38;5;241;43m433\u001B[39;49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[38;5;66;43;03m# Ensure this matches a valid sample ID in the database\u001B[39;49;00m\n\u001B[1;32m 23\u001B[0m \u001B[43m \u001B[49m\u001B[43msample_event_create\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43msample_event_create\u001B[49m\n\u001B[1;32m 24\u001B[0m \u001B[43m\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 26\u001B[0m \u001B[38;5;28mprint\u001B[39m(\u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mAPI response:\u001B[39m\u001B[38;5;124m\"\u001B[39m)\n\u001B[1;32m 27\u001B[0m pprint(api_response)\n",
|
|
"File \u001B[0;32m/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/pydantic/validate_call_decorator.py:60\u001B[0m, in \u001B[0;36mvalidate_call.<locals>.validate.<locals>.wrapper_function\u001B[0;34m(*args, **kwargs)\u001B[0m\n\u001B[1;32m 58\u001B[0m \u001B[38;5;129m@functools\u001B[39m\u001B[38;5;241m.\u001B[39mwraps(function)\n\u001B[1;32m 59\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21mwrapper_function\u001B[39m(\u001B[38;5;241m*\u001B[39margs, \u001B[38;5;241m*\u001B[39m\u001B[38;5;241m*\u001B[39mkwargs):\n\u001B[0;32m---> 60\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[43mvalidate_call_wrapper\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[43margs\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[43mkwargs\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/pydantic/_internal/_validate_call.py:96\u001B[0m, in \u001B[0;36mValidateCallWrapper.__call__\u001B[0;34m(self, *args, **kwargs)\u001B[0m\n\u001B[1;32m 95\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21m__call__\u001B[39m(\u001B[38;5;28mself\u001B[39m, \u001B[38;5;241m*\u001B[39margs: Any, \u001B[38;5;241m*\u001B[39m\u001B[38;5;241m*\u001B[39mkwargs: Any) \u001B[38;5;241m-\u001B[39m\u001B[38;5;241m>\u001B[39m Any:\n\u001B[0;32m---> 96\u001B[0m res \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m__pydantic_validator__\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mvalidate_python\u001B[49m\u001B[43m(\u001B[49m\u001B[43mpydantic_core\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mArgsKwargs\u001B[49m\u001B[43m(\u001B[49m\u001B[43margs\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mkwargs\u001B[49m\u001B[43m)\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 97\u001B[0m \u001B[38;5;28;01mif\u001B[39;00m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39m__return_pydantic_validator__:\n\u001B[1;32m 98\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39m__return_pydantic_validator__(res)\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api/samples_api.py:109\u001B[0m, in \u001B[0;36mSamplesApi.create_sample_event_samples_samples_sample_id_events_post\u001B[0;34m(self, sample_id, sample_event_create, _request_timeout, _request_auth, _content_type, _headers, _host_index)\u001B[0m\n\u001B[1;32m 104\u001B[0m response_data \u001B[38;5;241m=\u001B[39m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39mapi_client\u001B[38;5;241m.\u001B[39mcall_api(\n\u001B[1;32m 105\u001B[0m \u001B[38;5;241m*\u001B[39m_param,\n\u001B[1;32m 106\u001B[0m _request_timeout\u001B[38;5;241m=\u001B[39m_request_timeout\n\u001B[1;32m 107\u001B[0m )\n\u001B[1;32m 108\u001B[0m response_data\u001B[38;5;241m.\u001B[39mread()\n\u001B[0;32m--> 109\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mapi_client\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mresponse_deserialize\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 110\u001B[0m \u001B[43m \u001B[49m\u001B[43mresponse_data\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43mresponse_data\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 111\u001B[0m \u001B[43m \u001B[49m\u001B[43mresponse_types_map\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_response_types_map\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 112\u001B[0m \u001B[43m\u001B[49m\u001B[43m)\u001B[49m\u001B[38;5;241m.\u001B[39mdata\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:319\u001B[0m, in \u001B[0;36mApiClient.response_deserialize\u001B[0;34m(self, response_data, response_types_map)\u001B[0m\n\u001B[1;32m 317\u001B[0m encoding \u001B[38;5;241m=\u001B[39m match\u001B[38;5;241m.\u001B[39mgroup(\u001B[38;5;241m1\u001B[39m) \u001B[38;5;28;01mif\u001B[39;00m match \u001B[38;5;28;01melse\u001B[39;00m \u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mutf-8\u001B[39m\u001B[38;5;124m\"\u001B[39m\n\u001B[1;32m 318\u001B[0m response_text \u001B[38;5;241m=\u001B[39m response_data\u001B[38;5;241m.\u001B[39mdata\u001B[38;5;241m.\u001B[39mdecode(encoding)\n\u001B[0;32m--> 319\u001B[0m return_data \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mdeserialize\u001B[49m\u001B[43m(\u001B[49m\u001B[43mresponse_text\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mresponse_type\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mcontent_type\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 320\u001B[0m \u001B[38;5;28;01mfinally\u001B[39;00m:\n\u001B[1;32m 321\u001B[0m \u001B[38;5;28;01mif\u001B[39;00m \u001B[38;5;129;01mnot\u001B[39;00m \u001B[38;5;241m200\u001B[39m \u001B[38;5;241m<\u001B[39m\u001B[38;5;241m=\u001B[39m response_data\u001B[38;5;241m.\u001B[39mstatus \u001B[38;5;241m<\u001B[39m\u001B[38;5;241m=\u001B[39m \u001B[38;5;241m299\u001B[39m:\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:420\u001B[0m, in \u001B[0;36mApiClient.deserialize\u001B[0;34m(self, response_text, response_type, content_type)\u001B[0m\n\u001B[1;32m 414\u001B[0m \u001B[38;5;28;01melse\u001B[39;00m:\n\u001B[1;32m 415\u001B[0m \u001B[38;5;28;01mraise\u001B[39;00m ApiException(\n\u001B[1;32m 416\u001B[0m status\u001B[38;5;241m=\u001B[39m\u001B[38;5;241m0\u001B[39m,\n\u001B[1;32m 417\u001B[0m reason\u001B[38;5;241m=\u001B[39m\u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mUnsupported content type: \u001B[39m\u001B[38;5;132;01m{0}\u001B[39;00m\u001B[38;5;124m\"\u001B[39m\u001B[38;5;241m.\u001B[39mformat(content_type)\n\u001B[1;32m 418\u001B[0m )\n\u001B[0;32m--> 420\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m__deserialize\u001B[49m\u001B[43m(\u001B[49m\u001B[43mdata\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mresponse_type\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:467\u001B[0m, in \u001B[0;36mApiClient.__deserialize\u001B[0;34m(self, data, klass)\u001B[0m\n\u001B[1;32m 465\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39m__deserialize_enum(data, klass)\n\u001B[1;32m 466\u001B[0m \u001B[38;5;28;01melse\u001B[39;00m:\n\u001B[0;32m--> 467\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m__deserialize_model\u001B[49m\u001B[43m(\u001B[49m\u001B[43mdata\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mklass\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:788\u001B[0m, in \u001B[0;36mApiClient.__deserialize_model\u001B[0;34m(self, data, klass)\u001B[0m\n\u001B[1;32m 780\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21m__deserialize_model\u001B[39m(\u001B[38;5;28mself\u001B[39m, data, klass):\n\u001B[1;32m 781\u001B[0m \u001B[38;5;250m \u001B[39m\u001B[38;5;124;03m\"\"\"Deserializes list or dict to model.\u001B[39;00m\n\u001B[1;32m 782\u001B[0m \n\u001B[1;32m 783\u001B[0m \u001B[38;5;124;03m :param data: dict, list.\u001B[39;00m\n\u001B[1;32m 784\u001B[0m \u001B[38;5;124;03m :param klass: class literal.\u001B[39;00m\n\u001B[1;32m 785\u001B[0m \u001B[38;5;124;03m :return: model object.\u001B[39;00m\n\u001B[1;32m 786\u001B[0m \u001B[38;5;124;03m \"\"\"\u001B[39;00m\n\u001B[0;32m--> 788\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[43mklass\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mfrom_dict\u001B[49m\u001B[43m(\u001B[49m\u001B[43mdata\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/models/sample_event_response.py:86\u001B[0m, in \u001B[0;36mSampleEventResponse.from_dict\u001B[0;34m(cls, obj)\u001B[0m\n\u001B[1;32m 83\u001B[0m \u001B[38;5;28;01mif\u001B[39;00m \u001B[38;5;129;01mnot\u001B[39;00m \u001B[38;5;28misinstance\u001B[39m(obj, \u001B[38;5;28mdict\u001B[39m):\n\u001B[1;32m 84\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28mcls\u001B[39m\u001B[38;5;241m.\u001B[39mmodel_validate(obj)\n\u001B[0;32m---> 86\u001B[0m _obj \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;43mcls\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mmodel_validate\u001B[49m\u001B[43m(\u001B[49m\u001B[43m{\u001B[49m\n\u001B[1;32m 87\u001B[0m \u001B[43m \u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mid\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m:\u001B[49m\u001B[43m \u001B[49m\u001B[43mobj\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mget\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mid\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m)\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 88\u001B[0m \u001B[43m \u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43msample_id\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m:\u001B[49m\u001B[43m \u001B[49m\u001B[43mobj\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mget\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43msample_id\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m)\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 89\u001B[0m \u001B[43m \u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mevent_type\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m:\u001B[49m\u001B[43m \u001B[49m\u001B[43mobj\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mget\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mevent_type\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m)\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 90\u001B[0m \u001B[43m \u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mtimestamp\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m:\u001B[49m\u001B[43m \u001B[49m\u001B[43mobj\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mget\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[38;5;124;43mtimestamp\u001B[39;49m\u001B[38;5;124;43m\"\u001B[39;49m\u001B[43m)\u001B[49m\n\u001B[1;32m 91\u001B[0m \u001B[43m\u001B[49m\u001B[43m}\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 92\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m _obj\n",
|
|
"File \u001B[0;32m/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/pydantic/main.py:596\u001B[0m, in \u001B[0;36mBaseModel.model_validate\u001B[0;34m(cls, obj, strict, from_attributes, context)\u001B[0m\n\u001B[1;32m 594\u001B[0m \u001B[38;5;66;03m# `__tracebackhide__` tells pytest and some other tools to omit this function from tracebacks\u001B[39;00m\n\u001B[1;32m 595\u001B[0m __tracebackhide__ \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;01mTrue\u001B[39;00m\n\u001B[0;32m--> 596\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28;43mcls\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m__pydantic_validator__\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mvalidate_python\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 597\u001B[0m \u001B[43m \u001B[49m\u001B[43mobj\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mstrict\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43mstrict\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mfrom_attributes\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43mfrom_attributes\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mcontext\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43mcontext\u001B[49m\n\u001B[1;32m 598\u001B[0m \u001B[43m\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"\u001B[0;31mValidationError\u001B[0m: 3 validation errors for SampleEventResponse\nsample_id\n Input should be a valid integer [type=int_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/int_type\nevent_type\n Input should be a valid string [type=string_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/string_type\ntimestamp\n Input should be a valid datetime [type=datetime_type, input_value=None, input_type=NoneType]\n For further information visit https://errors.pydantic.dev/2.9/v/datetime_type"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 110
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-16T19:45:46.332149Z",
|
|
"start_time": "2025-01-16T19:45:46.320963Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the Samples API class\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Get the last sample event\n",
|
|
" last_event_response = api_instance.get_last_sample_event_samples_samples_sample_id_events_last_get(14)\n",
|
|
" print(\"The response of get_last_sample_event:\\n\")\n",
|
|
" pprint(last_event_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling get_last_sample_event: %s\\n\" % e)\n"
|
|
],
|
|
"id": "6a808ee09f97ae13",
|
|
"outputs": [
|
|
{
|
|
"ename": "NameError",
|
|
"evalue": "name 'aareDBclient' is not defined",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mNameError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[7], line 1\u001B[0m\n\u001B[0;32m----> 1\u001B[0m \u001B[38;5;28;01mwith\u001B[39;00m \u001B[43maareDBclient\u001B[49m\u001B[38;5;241m.\u001B[39mApiClient(configuration) \u001B[38;5;28;01mas\u001B[39;00m api_client:\n\u001B[1;32m 2\u001B[0m \u001B[38;5;66;03m# Create an instance of the Samples API class\u001B[39;00m\n\u001B[1;32m 3\u001B[0m api_instance \u001B[38;5;241m=\u001B[39m aareDBclient\u001B[38;5;241m.\u001B[39mSamplesApi(api_client)\n\u001B[1;32m 5\u001B[0m \u001B[38;5;28;01mtry\u001B[39;00m:\n\u001B[1;32m 6\u001B[0m \u001B[38;5;66;03m# Get the last sample event\u001B[39;00m\n",
|
|
"\u001B[0;31mNameError\u001B[0m: name 'aareDBclient' is not defined"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 7
|
|
}
|
|
],
|
|
"metadata": {
|
|
"kernelspec": {
|
|
"display_name": "Python 3",
|
|
"language": "python",
|
|
"name": "python3"
|
|
},
|
|
"language_info": {
|
|
"codemirror_mode": {
|
|
"name": "ipython",
|
|
"version": 2
|
|
},
|
|
"file_extension": ".py",
|
|
"mimetype": "text/x-python",
|
|
"name": "python",
|
|
"nbconvert_exporter": "python",
|
|
"pygments_lexer": "ipython2",
|
|
"version": "2.7.6"
|
|
}
|
|
},
|
|
"nbformat": 4,
|
|
"nbformat_minor": 5
|
|
}
|