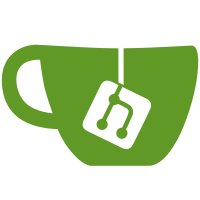
Upgraded multiple dependencies across Babel, Emotion, ESLint, and DevExpress packages in `package-lock.json` to their latest versions. These updates ensure compatibility, fix minor issues, and improve overall performance and security.
517 lines
23 KiB
Plaintext
517 lines
23 KiB
Plaintext
{
|
|
"cells": [
|
|
{
|
|
"cell_type": "code",
|
|
"id": "initial_id",
|
|
"metadata": {
|
|
"collapsed": true,
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:03:52.460891Z",
|
|
"start_time": "2025-01-17T14:03:52.454842Z"
|
|
}
|
|
},
|
|
"source": [
|
|
"import json\n",
|
|
"import backend.aareDBclient as aareDBclient\n",
|
|
"from aareDBclient.rest import ApiException\n",
|
|
"from pprint import pprint\n",
|
|
"#from aareDBclient import SamplesApi, ShipmentsApi, PucksApi\n",
|
|
"#from aareDBclient.models import SampleEventCreate, SetTellPosition\n",
|
|
"#from examples.examples import api_response\n",
|
|
"\n",
|
|
"print(aareDBclient.__version__)\n",
|
|
"\n",
|
|
"configuration = aareDBclient.Configuration(\n",
|
|
" #host = \"https://mx-aare-test.psi.ch:1492\"\n",
|
|
" host = \"https://127.0.0.1:8000\"\n",
|
|
")\n",
|
|
"\n",
|
|
"print(configuration.host)\n",
|
|
"\n",
|
|
"configuration.verify_ssl = False # Disable SSL verification\n",
|
|
"#print(dir(SamplesApi))"
|
|
],
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"0.1.0a19\n",
|
|
"https://127.0.0.1:8000\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 40
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:13:16.559702Z",
|
|
"start_time": "2025-01-17T14:13:16.446021Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"## Fetch all Shipments, list corresponding dewars and pucks\n",
|
|
"\n",
|
|
"from datetime import date\n",
|
|
"from aareDBclient import ShipmentsApi\n",
|
|
"from aareDBclient.models import Shipment\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.ShipmentsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Fetch all shipments\n",
|
|
" all_shipments_response = api_instance.fetch_shipments_shipments_get()\n",
|
|
"\n",
|
|
" # Print shipment names and their associated puck names\n",
|
|
" for shipment in all_shipments_response:\n",
|
|
" print(f\"Shipment ID: {shipment.id}, Shipment Name: {shipment.shipment_name}\")\n",
|
|
" if hasattr(shipment, 'dewars') and shipment.dewars: # Ensure 'dewars' exists\n",
|
|
" for dewar in shipment.dewars:\n",
|
|
" print(f\" Dewar ID: {dewar.id}, Dewar Name: {dewar.dewar_name}, Dewar Unique ID: {dewar.unique_id} \")\n",
|
|
"\n",
|
|
" if hasattr(dewar, 'pucks') and dewar.pucks: # Ensure 'pucks' exists\n",
|
|
" for puck in dewar.pucks:\n",
|
|
" print(f\" Puck ID: {puck.id}, Puck Name: {puck.puck_name}\")\n",
|
|
" else:\n",
|
|
" print(\" No pucks found in this dewar.\")\n",
|
|
" else:\n",
|
|
" print(\" No dewars found in this shipment.\")\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling ShipmentsApi->fetch_shipments_shipments_get: {e}\")\n"
|
|
],
|
|
"id": "45cc7ab6d4589711",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Shipment ID: 2, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 1, Dewar Name: Dewar One, Dewar Unique ID: 83041c3f8844ff39 \n",
|
|
" Puck ID: 1, Puck Name: PUCK-001\n",
|
|
" Puck ID: 2, Puck Name: PUCK002\n",
|
|
" Puck ID: 3, Puck Name: PUCK003\n",
|
|
" Puck ID: 4, Puck Name: PUCK004\n",
|
|
" Puck ID: 5, Puck Name: PUCK005\n",
|
|
" Puck ID: 6, Puck Name: PUCK006\n",
|
|
" Puck ID: 7, Puck Name: PUCK007\n",
|
|
" Dewar ID: 2, Dewar Name: Dewar Two, Dewar Unique ID: 62baccecdd37c033 \n",
|
|
" Puck ID: 8, Puck Name: PK001\n",
|
|
" Puck ID: 9, Puck Name: PK002\n",
|
|
" Puck ID: 10, Puck Name: PK003\n",
|
|
" Puck ID: 11, Puck Name: PK004\n",
|
|
" Puck ID: 12, Puck Name: PK005\n",
|
|
" Puck ID: 13, Puck Name: PK006\n",
|
|
"Shipment ID: 3, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 3, Dewar Name: Dewar Three, Dewar Unique ID: None \n",
|
|
" Puck ID: 14, Puck Name: P001\n",
|
|
" Puck ID: 15, Puck Name: P002\n",
|
|
" Puck ID: 16, Puck Name: P003\n",
|
|
" Puck ID: 17, Puck Name: P004\n",
|
|
" Puck ID: 18, Puck Name: P005\n",
|
|
" Puck ID: 19, Puck Name: P006\n",
|
|
" Puck ID: 20, Puck Name: P007\n",
|
|
" Dewar ID: 4, Dewar Name: Dewar Four, Dewar Unique ID: None \n",
|
|
" Puck ID: 21, Puck Name: PC002\n",
|
|
" Puck ID: 22, Puck Name: PC003\n",
|
|
" Puck ID: 23, Puck Name: PC004\n",
|
|
" Puck ID: 24, Puck Name: PC005\n",
|
|
" Puck ID: 25, Puck Name: PC006\n",
|
|
" Puck ID: 26, Puck Name: PC007\n",
|
|
"Shipment ID: 1, Shipment Name: Shipment from Mordor\n",
|
|
" Dewar ID: 5, Dewar Name: Dewar Five, Dewar Unique ID: 15e3dbe05e78ee83 \n",
|
|
" Puck ID: 27, Puck Name: PKK004\n",
|
|
" Puck ID: 28, Puck Name: PKK005\n",
|
|
" Puck ID: 29, Puck Name: PKK006\n",
|
|
" Puck ID: 30, Puck Name: PKK007\n",
|
|
"Shipment ID: 4, Shipment Name: testship\n",
|
|
" Dewar ID: 6, Dewar Name: Dewar_test, Dewar Unique ID: f352529444d64dd5 \n",
|
|
" Puck ID: 43, Puck Name: CPS-4093\n",
|
|
" Puck ID: 44, Puck Name: CPS-4178\n",
|
|
" Puck ID: 45, Puck Name: PSIMX-122\n",
|
|
" Puck ID: 46, Puck Name: CPS-6597\n",
|
|
" Puck ID: 47, Puck Name: PSIMX-078\n",
|
|
" Puck ID: 48, Puck Name: 1002\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 44
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:14:30.201369Z",
|
|
"start_time": "2025-01-17T14:14:30.175577Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from datetime import date\n",
|
|
"from aareDBclient import LogisticsApi\n",
|
|
"from aareDBclient.models import LogisticsEventCreate # Import required model\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.LogisticsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='incoming',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='refill',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='X06DA-Beamline',\n",
|
|
" transaction_type='beamline',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n"
|
|
],
|
|
"id": "f5de1787214a6642",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 45
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:07:51.580993Z",
|
|
"start_time": "2025-01-17T14:07:51.565128Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Get a list of pucks that are \"at the beamline\"\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create Return Address\n",
|
|
" api_response = api_instance.get_pucks_by_slot_pucks_slot_slot_identifier_get(slot_identifier='X06DA')\n",
|
|
" print(\"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get: %s\\n\" % e)"
|
|
],
|
|
"id": "bbee7c94bf14000c",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\n",
|
|
"\n",
|
|
"[PuckWithTellPosition(id=43, puck_name='CPS-4093', puck_type='unipuck', puck_location_in_dewar=1, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=44, puck_name='CPS-4178', puck_type='unipuck', puck_location_in_dewar=2, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=45, puck_name='PSIMX-122', puck_type='unipuck', puck_location_in_dewar=3, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=46, puck_name='CPS-6597', puck_type='unipuck', puck_location_in_dewar=4, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=47, puck_name='PSIMX-078', puck_type='unipuck', puck_location_in_dewar=5, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=48, puck_name='1002', puck_type='unipuck', puck_location_in_dewar=6, dewar_id=6, dewar_name='Dewar_test', user='e16371', samples=None, tell_position=None)]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 42
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:09:55.141237Z",
|
|
"start_time": "2025-01-17T14:09:55.117843Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Attribute a puck to a position in the TELL dewar\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # This part is commented but will be used to attribute a puck to a position of the TELL\n",
|
|
" # Define the puck ID and payload\n",
|
|
"\n",
|
|
" payload = [SetTellPosition(puck_name='CPS-4178', segment='A', puck_in_segment=2),SetTellPosition(puck_name='PSIMX122', segment='C', puck_in_segment=3)]\n",
|
|
" #payload = []\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Call the PUT method to update the tell_position\n",
|
|
" api_response = api_instance.set_tell_positions_pucks_set_tell_positions_put(payload)\n",
|
|
" print(\"The response of PucksApi->pucks_puck_id_tell_position_put:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->pucks_puck_id_tell_position_put: %s\\n\" % e)"
|
|
],
|
|
"id": "d52d12287dd63299",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->pucks_puck_id_tell_position_put:\n",
|
|
"\n",
|
|
"[{'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'A2',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'CPS-4178',\n",
|
|
" 'status': 'updated'},\n",
|
|
" {'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'C3',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'PSIMX-122',\n",
|
|
" 'status': 'updated'}]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 43
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-16T19:45:40.811948Z",
|
|
"start_time": "2025-01-16T19:45:40.798389Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # GET request: Fetch all pucks in the tell\n",
|
|
" try:\n",
|
|
" all_pucks_response = api_instance.get_pucks_with_tell_position_pucks_with_tell_position_get() # Replace with appropriate method\n",
|
|
" # Convert the response to a JSON-like string for pretty printing\n",
|
|
" formatted_response = json.dumps(\n",
|
|
" [puck.to_dict() for puck in all_pucks_response], # .to_dict() assumes API models can convert to Python dict\n",
|
|
" indent=4 # Use indentation for readability\n",
|
|
" )\n",
|
|
"\n",
|
|
" print(\"The response of PucksApi->get_all_pucks_in_tell:\\n\")\n",
|
|
" #print(formatted_response)\n",
|
|
" for p in all_pucks_response:\n",
|
|
" print(p.puck_name)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_all_pucks_in_tell: %s\\n\" % e)"
|
|
],
|
|
"id": "95f8c133359945d5",
|
|
"outputs": [
|
|
{
|
|
"ename": "NameError",
|
|
"evalue": "name 'aareDBclient' is not defined",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mNameError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[5], line 1\u001B[0m\n\u001B[0;32m----> 1\u001B[0m \u001B[38;5;28;01mwith\u001B[39;00m \u001B[43maareDBclient\u001B[49m\u001B[38;5;241m.\u001B[39mApiClient(configuration) \u001B[38;5;28;01mas\u001B[39;00m api_client:\n\u001B[1;32m 2\u001B[0m \u001B[38;5;66;03m# Create an instance of the API class\u001B[39;00m\n\u001B[1;32m 3\u001B[0m api_instance \u001B[38;5;241m=\u001B[39m aareDBclient\u001B[38;5;241m.\u001B[39mPucksApi(api_client)\n\u001B[1;32m 5\u001B[0m \u001B[38;5;66;03m# GET request: Fetch all pucks in the tell\u001B[39;00m\n",
|
|
"\u001B[0;31mNameError\u001B[0m: name 'aareDBclient' is not defined"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 5
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-16T19:45:44.042534Z",
|
|
"start_time": "2025-01-16T19:45:44.030292Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the Samples API class\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" # Define the sample ID and event payload using the expected model\n",
|
|
" sample_id = 261\n",
|
|
" event_payload = SampleEventCreate(\n",
|
|
" event_type=\"Failed\" # Replace with actual event type if different\n",
|
|
" )\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Post the sample event\n",
|
|
" api_response = api_instance.create_sample_event_samples_samples_sample_id_events_post(\n",
|
|
" sample_id=sample_id,\n",
|
|
" sample_event_create=event_payload # Pass the model instance here\n",
|
|
" )\n",
|
|
" print(\"The response of post_sample_event:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling post_sample_event: %s\\n\" % e)\n"
|
|
],
|
|
"id": "ee8abb293096334a",
|
|
"outputs": [
|
|
{
|
|
"ename": "NameError",
|
|
"evalue": "name 'aareDBclient' is not defined",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mNameError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[6], line 1\u001B[0m\n\u001B[0;32m----> 1\u001B[0m \u001B[38;5;28;01mwith\u001B[39;00m \u001B[43maareDBclient\u001B[49m\u001B[38;5;241m.\u001B[39mApiClient(configuration) \u001B[38;5;28;01mas\u001B[39;00m api_client:\n\u001B[1;32m 2\u001B[0m \u001B[38;5;66;03m# Create an instance of the Samples API class\u001B[39;00m\n\u001B[1;32m 3\u001B[0m api_instance \u001B[38;5;241m=\u001B[39m aareDBclient\u001B[38;5;241m.\u001B[39mSamplesApi(api_client)\n\u001B[1;32m 5\u001B[0m \u001B[38;5;66;03m# Define the sample ID and event payload using the expected model\u001B[39;00m\n",
|
|
"\u001B[0;31mNameError\u001B[0m: name 'aareDBclient' is not defined"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 6
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-16T19:45:46.332149Z",
|
|
"start_time": "2025-01-16T19:45:46.320963Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the Samples API class\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Get the last sample event\n",
|
|
" last_event_response = api_instance.get_last_sample_event_samples_samples_sample_id_events_last_get(14)\n",
|
|
" print(\"The response of get_last_sample_event:\\n\")\n",
|
|
" pprint(last_event_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling get_last_sample_event: %s\\n\" % e)\n"
|
|
],
|
|
"id": "6a808ee09f97ae13",
|
|
"outputs": [
|
|
{
|
|
"ename": "NameError",
|
|
"evalue": "name 'aareDBclient' is not defined",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mNameError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[7], line 1\u001B[0m\n\u001B[0;32m----> 1\u001B[0m \u001B[38;5;28;01mwith\u001B[39;00m \u001B[43maareDBclient\u001B[49m\u001B[38;5;241m.\u001B[39mApiClient(configuration) \u001B[38;5;28;01mas\u001B[39;00m api_client:\n\u001B[1;32m 2\u001B[0m \u001B[38;5;66;03m# Create an instance of the Samples API class\u001B[39;00m\n\u001B[1;32m 3\u001B[0m api_instance \u001B[38;5;241m=\u001B[39m aareDBclient\u001B[38;5;241m.\u001B[39mSamplesApi(api_client)\n\u001B[1;32m 5\u001B[0m \u001B[38;5;28;01mtry\u001B[39;00m:\n\u001B[1;32m 6\u001B[0m \u001B[38;5;66;03m# Get the last sample event\u001B[39;00m\n",
|
|
"\u001B[0;31mNameError\u001B[0m: name 'aareDBclient' is not defined"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 7
|
|
}
|
|
],
|
|
"metadata": {
|
|
"kernelspec": {
|
|
"display_name": "Python 3",
|
|
"language": "python",
|
|
"name": "python3"
|
|
},
|
|
"language_info": {
|
|
"codemirror_mode": {
|
|
"name": "ipython",
|
|
"version": 2
|
|
},
|
|
"file_extension": ".py",
|
|
"mimetype": "text/x-python",
|
|
"name": "python",
|
|
"nbconvert_exporter": "python",
|
|
"pygments_lexer": "ipython2",
|
|
"version": "2.7.6"
|
|
}
|
|
},
|
|
"nbformat": 4,
|
|
"nbformat_minor": 5
|
|
}
|