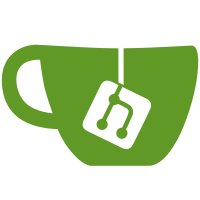
Updated several frontend dependencies including MUI packages and added new ones like `@mui/x-charts`. Adjusted the Python path setup in the CI configuration to correctly point to the `aaredb` backend, ensuring accurate module resolution.
45 lines
1.3 KiB
Python
45 lines
1.3 KiB
Python
# tests/test_auth.py
|
|
|
|
import pytest
|
|
from fastapi.testclient import TestClient
|
|
from backend.main import app
|
|
|
|
|
|
@pytest.fixture(scope="module")
|
|
def client():
|
|
with TestClient(app) as test_client: # ensures lifespan/startup executes
|
|
yield test_client
|
|
|
|
|
|
def test_login_success(client):
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "testuser", "password": "testpass"}
|
|
)
|
|
assert response.status_code == 200
|
|
assert "access_token" in response.json()
|
|
|
|
|
|
def test_login_failure(client):
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "wrong", "password": "wrongpass"}
|
|
)
|
|
assert response.status_code == 401
|
|
assert response.json() == {"detail": "Incorrect username or password"}
|
|
|
|
|
|
def test_protected_route(client):
|
|
# Step 1: Login
|
|
response = client.post(
|
|
"/auth/token/login", data={"username": "testuser", "password": "testpass"}
|
|
)
|
|
token = response.json()["access_token"]
|
|
|
|
# Step 2: Access protected route
|
|
headers = {"Authorization": f"Bearer {token}"}
|
|
response = client.get("/auth/protected-route", headers=headers)
|
|
assert response.status_code == 200
|
|
assert response.json() == {
|
|
"username": "testuser",
|
|
"pgroups": ["p20000", "p20001", "p20002", "p20003"],
|
|
}
|