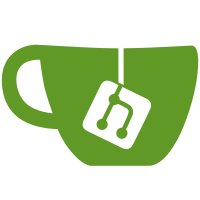
This script demonstrates various AareDB client API operations, including retrieving pucks, posting sample events, and fetching the last sample event. It serves as a reference for interacting with the AareDB system using Python.
102 lines
3.6 KiB
Python
102 lines
3.6 KiB
Python
# flake8: noqa: E501
|
|
import aareDBclient
|
|
from aareDBclient.rest import ApiException
|
|
from pprint import pprint
|
|
from aareDBclient import SamplesApi
|
|
from aareDBclient.models import SampleEventCreate
|
|
|
|
|
|
print(aareDBclient.__version__)
|
|
|
|
# Defining the host is optional and defaults to http://localhost
|
|
# See configuration.py for a list of all supported configuration parameters.
|
|
configuration = aareDBclient.Configuration(host="https://mx-aare-test.psi.ch:1492")
|
|
|
|
configuration.verify_ssl = False # Disable SSL verification
|
|
print(dir(SamplesApi))
|
|
|
|
# Enter a context with an instance of the API client
|
|
with aareDBclient.ApiClient(configuration) as api_client:
|
|
# Create an instance of the API class
|
|
api_instance = aareDBclient.PucksApi(api_client)
|
|
get_pucks_at_beamline = aareDBclient.PucksApi(api_client)
|
|
|
|
try:
|
|
# Create Return Address
|
|
api_response = api_instance.get_pucks_by_slot_pucks_slot_slot_identifier_get(
|
|
slot_identifier="X06DA"
|
|
)
|
|
print(
|
|
"The response of PucksApi->"
|
|
"get_pucks_by_slot_pucks_slot_slot_identifier_get:\n"
|
|
)
|
|
pprint(api_response)
|
|
|
|
except ApiException as e:
|
|
print(
|
|
"Exception when calling PucksApi->"
|
|
"get_pucks_by_slot_pucks_slot_slot_identifier_get: %s\n" % e
|
|
)
|
|
|
|
# This part is commented but will be used to attribute a puck to a position of the TELL
|
|
# Define the puck ID and payload
|
|
# puck_id = 1 # Equivalent to /pucks/6
|
|
# tell_position_payload = {"tell_position": "B1"}
|
|
|
|
# try:
|
|
# # Call the PUT method to update the tell_position
|
|
# api_response = api_instance.set_tell_position_pucks_puck_id_tell_position_put(
|
|
# puck_id=puck_id,
|
|
# set_tell_position=tell_position_payload)
|
|
# print("The response of PucksApi->pucks_puck_id_tell_position_put:\n")
|
|
# pprint(api_response)
|
|
#
|
|
# except ApiException as e:
|
|
# print("Exception when calling PucksApi->pucks_puck_id_tell_position_put: %s\n" % e)
|
|
|
|
# GET request: Fetch all pucks in the tell
|
|
try:
|
|
all_pucks_response = (
|
|
api_instance.get_pucks_with_tell_position_pucks_with_tell_position_get()
|
|
) # Replace with appropriate method
|
|
print("The response of PucksApi->get_all_pucks_in_tell:\n")
|
|
pprint(all_pucks_response)
|
|
|
|
except ApiException as e:
|
|
print("Exception when calling PucksApi->get_all_pucks_in_tell: %s\n" % e)
|
|
|
|
with aareDBclient.ApiClient(configuration) as api_client:
|
|
# Create an instance of the Samples API class
|
|
api_instance = aareDBclient.SamplesApi(api_client)
|
|
|
|
# Define the sample ID and event payload using the expected model
|
|
sample_id = 1
|
|
event_payload = SampleEventCreate(
|
|
event_type="Unmounted" # Replace with actual event type if different
|
|
)
|
|
|
|
try:
|
|
# Post the sample event
|
|
api_response = (
|
|
api_instance.create_sample_event_samples_samples_sample_id_events_post(
|
|
sample_id=sample_id,
|
|
sample_event_create=event_payload, # Pass the model instance here
|
|
)
|
|
)
|
|
print("The response of post_sample_event:\n")
|
|
pprint(api_response)
|
|
|
|
except ApiException as e:
|
|
print("Exception when calling post_sample_event: %s\n" % e)
|
|
|
|
try:
|
|
# Get the last sample event
|
|
last_event_response = api_instance.get_last_sample_event_samples_samples_sample_id_events_last_get(
|
|
1
|
|
)
|
|
print("The response of get_last_sample_event:\n")
|
|
pprint(last_event_response)
|
|
|
|
except ApiException as e:
|
|
print("Exception when calling get_last_sample_event: %s\n" % e)
|