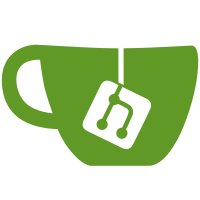
Updated Dewar API methods to use protected endpoints for enhanced security and consistency. Added `pgroups` handling in various frontend components and modified the LogisticsView contact field for clarity. Simplified backend router imports for better readability.
660 lines
42 KiB
Plaintext
660 lines
42 KiB
Plaintext
{
|
|
"cells": [
|
|
{
|
|
"cell_type": "code",
|
|
"id": "initial_id",
|
|
"metadata": {
|
|
"collapsed": true,
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T11:29:38.703954Z",
|
|
"start_time": "2025-01-30T11:29:38.307050Z"
|
|
}
|
|
},
|
|
"source": [
|
|
"import json\n",
|
|
"\n",
|
|
"#from nbclient.client import timestamp\n",
|
|
"\n",
|
|
"import backend.aareDBclient as aareDBclient\n",
|
|
"from aareDBclient.rest import ApiException\n",
|
|
"from pprint import pprint\n",
|
|
"\n",
|
|
"#from app.data.data import sample\n",
|
|
"\n",
|
|
"#from aareDBclient import SamplesApi, ShipmentsApi, PucksApi\n",
|
|
"#from aareDBclient.models import SampleEventCreate, SetTellPosition\n",
|
|
"#from examples.examples import api_response\n",
|
|
"\n",
|
|
"print(aareDBclient.__version__)\n",
|
|
"\n",
|
|
"configuration = aareDBclient.Configuration(\n",
|
|
" #host = \"https://mx-aare-test.psi.ch:1492\"\n",
|
|
" host = \"https://127.0.0.1:8000\"\n",
|
|
")\n",
|
|
"\n",
|
|
"print(configuration.host)\n",
|
|
"\n",
|
|
"configuration.verify_ssl = False # Disable SSL verification\n",
|
|
"#print(dir(SamplesApi))"
|
|
],
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"0.1.0a20\n",
|
|
"https://127.0.0.1:8000\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 1
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T11:07:14.795059Z",
|
|
"start_time": "2025-01-30T11:07:14.783786Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"## Fetch all Shipments, list corresponding dewars and pucks\n",
|
|
"\n",
|
|
"from datetime import date, datetime\n",
|
|
"from aareDBclient import ShipmentsApi\n",
|
|
"from aareDBclient.models import Shipment\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.ShipmentsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Fetch all shipments\n",
|
|
" all_shipments_response = api_instance.fetch_shipments_shipments_get()\n",
|
|
"\n",
|
|
" # Print shipment names and their associated puck names\n",
|
|
" for shipment in all_shipments_response:\n",
|
|
" print(f\"Shipment ID: {shipment.id}, Shipment Name: {shipment.shipment_name}\")\n",
|
|
" if hasattr(shipment, 'dewars') and shipment.dewars: # Ensure 'dewars' exists\n",
|
|
" for dewar in shipment.dewars:\n",
|
|
" print(f\" Dewar ID: {dewar.id}, Dewar Name: {dewar.dewar_name}, Dewar Unique ID: {dewar.unique_id} \")\n",
|
|
"\n",
|
|
" if hasattr(dewar, 'pucks') and dewar.pucks: # Ensure 'pucks' exists\n",
|
|
" for puck in dewar.pucks:\n",
|
|
" print(f\" Puck ID: {puck.id}, Puck Name: {puck.puck_name}\")\n",
|
|
" else:\n",
|
|
" print(\" No pucks found in this dewar.\")\n",
|
|
" else:\n",
|
|
" print(\" No dewars found in this shipment.\")\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling ShipmentsApi->fetch_shipments_shipments_get: {e}\")\n"
|
|
],
|
|
"id": "45cc7ab6d4589711",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Exception when calling ShipmentsApi->fetch_shipments_shipments_get: (404)\n",
|
|
"Reason: Not Found\n",
|
|
"HTTP response headers: HTTPHeaderDict({'date': 'Thu, 30 Jan 2025 11:07:14 GMT', 'server': 'uvicorn', 'content-length': '22', 'content-type': 'application/json'})\n",
|
|
"HTTP response body: {\"detail\":\"Not Found\"}\n",
|
|
"\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 6
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-17T14:14:30.201369Z",
|
|
"start_time": "2025-01-17T14:14:30.175577Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from datetime import date\n",
|
|
"from aareDBclient import LogisticsApi\n",
|
|
"from aareDBclient.models import LogisticsEventCreate # Import required model\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" api_instance = aareDBclient.LogisticsApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='incoming',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='A2-X06SA',\n",
|
|
" transaction_type='refill',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create payload using the required model\n",
|
|
" logistics_event_create = LogisticsEventCreate(\n",
|
|
" dewar_qr_code='15e3dbe05e78ee83',\n",
|
|
" location_qr_code='X06DA-Beamline',\n",
|
|
" transaction_type='beamline',\n",
|
|
" timestamp=date.today() # Adjust if the API expects datetime\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Pass the payload to the API function\n",
|
|
" api_response = api_instance.scan_dewar_logistics_dewar_scan_post(\n",
|
|
" logistics_event_create=logistics_event_create # Pass as an object\n",
|
|
" )\n",
|
|
" print(\"API Response:\", api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(f\"Exception when calling LogisticsApi->scan_dewar_logistics_dewar_scan_post: {e}\")\n"
|
|
],
|
|
"id": "f5de1787214a6642",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n",
|
|
"API Response: {'message': 'Status updated successfully'}\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n",
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 45
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T11:35:20.036682Z",
|
|
"start_time": "2025-01-30T11:35:20.018284Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Get a list of pucks that are \"at the beamline\"\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Create Return Address\n",
|
|
" api_response = api_instance.get_pucks_by_slot_pucks_slot_slot_identifier_get(slot_identifier='X06DA')\n",
|
|
" print(\"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get: %s\\n\" % e)"
|
|
],
|
|
"id": "bbee7c94bf14000c",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->get_pucks_by_slot_pucks_slot_slot_identifier_get:\n",
|
|
"\n",
|
|
"[PuckWithTellPosition(id=1, puck_name='PUCK-001', puck_type='Unipuck', puck_location_in_dewar=1, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=2, puck_name='PUCK002', puck_type='Unipuck', puck_location_in_dewar=2, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=3, puck_name='PUCK003', puck_type='Unipuck', puck_location_in_dewar=3, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=4, puck_name='PUCK004', puck_type='Unipuck', puck_location_in_dewar=4, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=5, puck_name='PUCK005', puck_type='Unipuck', puck_location_in_dewar=5, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=6, puck_name='PUCK006', puck_type='Unipuck', puck_location_in_dewar=6, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None),\n",
|
|
" PuckWithTellPosition(id=7, puck_name='PUCK007', puck_type='Unipuck', puck_location_in_dewar=7, dewar_id=1, dewar_name='Dewar One', pgroup='p20001', samples=None, tell_position=None)]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 5
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T12:30:46.242711Z",
|
|
"start_time": "2025-01-30T12:30:46.215343Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from aareDBclient import SetTellPosition\n",
|
|
"\n",
|
|
"# Attribute a puck to a position in the TELL dewar\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
" get_pucks_at_beamline = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # This part is commented but will be used to attribute a puck to a position of the TELL\n",
|
|
" # Define the puck ID and payload\n",
|
|
"\n",
|
|
" payload = [SetTellPosition(puck_name='PUCK006', segment='A', puck_in_segment=2),SetTellPosition(puck_name='PUCK005', segment='C', puck_in_segment=3)]\n",
|
|
" #payload = []\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Call the PUT method to update the tell_position\n",
|
|
" api_response = api_instance.set_tell_positions_pucks_set_tell_positions_put(payload)\n",
|
|
" print(\"The response of PucksApi->pucks_puck_id_tell_position_put:\\n\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->pucks_puck_id_tell_position_put: %s\\n\" % e)"
|
|
],
|
|
"id": "d52d12287dd63299",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"The response of PucksApi->pucks_puck_id_tell_position_put:\n",
|
|
"\n",
|
|
"[{'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'A2',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'PUCK006',\n",
|
|
" 'status': 'updated'},\n",
|
|
" {'message': 'The tell_position was updated successfully.',\n",
|
|
" 'new_position': 'C3',\n",
|
|
" 'previous_position': None,\n",
|
|
" 'puck_name': 'PUCK005',\n",
|
|
" 'status': 'updated'}]\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 8
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T12:35:40.734188Z",
|
|
"start_time": "2025-01-30T12:35:40.679071Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"# Get puck_id puck_name sample_id sample_name of pucks in the tell\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the API class\n",
|
|
" api_instance = aareDBclient.PucksApi(api_client)\n",
|
|
"\n",
|
|
" # GET request: Fetch all pucks in the tell\n",
|
|
" try:\n",
|
|
" # Call the API method to fetch pucks\n",
|
|
" all_pucks_response = api_instance.get_pucks_with_tell_position_pucks_with_tell_position_get()\n",
|
|
"\n",
|
|
" # Debug response structure by printing it in JSON format\n",
|
|
" formatted_response = json.dumps(\n",
|
|
" [p.to_dict() for p in all_pucks_response], # Assuming the API response can be converted to dicts\n",
|
|
" indent=4 # Use indentation for readability\n",
|
|
" )\n",
|
|
" #print(\"The response of PucksApi->get_all_pucks_in_tell (formatted):\\n\")\n",
|
|
" #print(formatted_response)\n",
|
|
"\n",
|
|
" # Iterate through each puck and print information\n",
|
|
" for p in all_pucks_response:\n",
|
|
" print(f\"Puck ID: {p.id}, Puck Name: {p.puck_name}\")\n",
|
|
"\n",
|
|
" # Check if the puck has any samples\n",
|
|
" if hasattr(p, 'samples') and p.samples: # Ensure 'samples' attribute exists and is not empty\n",
|
|
" for sample in p.samples:\n",
|
|
" print(f\" Sample ID: {sample.id}, Sample Name: {sample.sample_name}\")\n",
|
|
" else:\n",
|
|
" print(\" No samples found in this puck.\")\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling PucksApi->get_all_pucks_in_tell: %s\\n\" % e)"
|
|
],
|
|
"id": "95f8c133359945d5",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Puck ID: 6, Puck Name: PUCK006\n",
|
|
" Sample ID: 28, Sample Name: Sample028\n",
|
|
" Sample ID: 29, Sample Name: Sample029\n",
|
|
" Sample ID: 30, Sample Name: Sample030\n",
|
|
" Sample ID: 31, Sample Name: Sample031\n",
|
|
" Sample ID: 32, Sample Name: Sample032\n",
|
|
" Sample ID: 33, Sample Name: Sample033\n",
|
|
" Sample ID: 34, Sample Name: Sample034\n",
|
|
" Sample ID: 35, Sample Name: Sample035\n",
|
|
" Sample ID: 36, Sample Name: Sample036\n",
|
|
" Sample ID: 37, Sample Name: Sample037\n",
|
|
" Sample ID: 38, Sample Name: Sample038\n",
|
|
" Sample ID: 39, Sample Name: Sample039\n",
|
|
" Sample ID: 40, Sample Name: Sample040\n",
|
|
"Puck ID: 5, Puck Name: PUCK005\n",
|
|
" Sample ID: 21, Sample Name: Sample021\n",
|
|
" Sample ID: 22, Sample Name: Sample022\n",
|
|
" Sample ID: 23, Sample Name: Sample023\n",
|
|
" Sample ID: 24, Sample Name: Sample024\n",
|
|
" Sample ID: 25, Sample Name: Sample025\n",
|
|
" Sample ID: 26, Sample Name: Sample026\n",
|
|
" Sample ID: 27, Sample Name: Sample027\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 10
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T12:36:50.600728Z",
|
|
"start_time": "2025-01-30T12:36:50.581752Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from aareDBclient import SampleEventCreate\n",
|
|
"\n",
|
|
"# Create an event for a sample\n",
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Instance of the API client\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Define the payload with only `event_type`\n",
|
|
" sample_event_create = SampleEventCreate(\n",
|
|
" sample_id=27,\n",
|
|
" event_type=\"Unmounted\" # Valid event type\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Debug the payload before sending\n",
|
|
" print(\"Payload being sent to API:\")\n",
|
|
" print(sample_event_create.json()) # Ensure it matches `SampleEventCreate`\n",
|
|
"\n",
|
|
" # Call the API\n",
|
|
" api_response = api_instance.create_sample_event_samples_samples_sample_id_events_post(\n",
|
|
" sample_id=27, # Ensure this matches a valid sample ID in the database\n",
|
|
" sample_event_create=sample_event_create\n",
|
|
" )\n",
|
|
"\n",
|
|
" print(\"API response:\")\n",
|
|
" pprint(api_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling post_sample_event:\")\n",
|
|
" print(f\"Status Code: {e.status}\")\n",
|
|
" if e.body:\n",
|
|
" print(f\"Error Details: {e.body}\")\n"
|
|
],
|
|
"id": "ee8abb293096334a",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Payload being sent to API:\n",
|
|
"{\"event_type\":\"Unmounted\"}\n",
|
|
"API response:\n",
|
|
"Sample(id=27, sample_name='Sample027', position=15, puck_id=5, crystalname=None, proteinname=None, positioninpuck=None, priority=None, comments=None, data_collection_parameters=None, events=[SampleEventResponse(id=406, sample_id=27, event_type='Mounted', timestamp=datetime.datetime(2025, 1, 30, 13, 36, 34)), SampleEventResponse(id=407, sample_id=27, event_type='Unmounted', timestamp=datetime.datetime(2025, 1, 30, 13, 36, 51))], mount_count=1, unmount_count=1)\n"
|
|
]
|
|
},
|
|
{
|
|
"name": "stderr",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/urllib3/connectionpool.py:1097: InsecureRequestWarning: Unverified HTTPS request is being made to host '127.0.0.1'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings\n",
|
|
" warnings.warn(\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 13
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T12:37:14.520342Z",
|
|
"start_time": "2025-01-30T12:37:14.508460Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"\n",
|
|
"with aareDBclient.ApiClient(configuration) as api_client:\n",
|
|
" # Create an instance of the Samples API class\n",
|
|
" api_instance = aareDBclient.SamplesApi(api_client)\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Get the last sample event\n",
|
|
" last_event_response = api_instance.get_last_sample_event_samples_samples_sample_id_events_last_get(14)\n",
|
|
" print(\"The response of get_last_sample_event:\\n\")\n",
|
|
" pprint(last_event_response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" print(\"Exception when calling get_last_sample_event: %s\\n\" % e)\n"
|
|
],
|
|
"id": "6a808ee09f97ae13",
|
|
"outputs": [
|
|
{
|
|
"ename": "AttributeError",
|
|
"evalue": "'SamplesApi' object has no attribute 'get_last_sample_event_samples_samples_sample_id_events_last_get'",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mAttributeError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[14], line 7\u001B[0m\n\u001B[1;32m 3\u001B[0m api_instance \u001B[38;5;241m=\u001B[39m aareDBclient\u001B[38;5;241m.\u001B[39mSamplesApi(api_client)\n\u001B[1;32m 5\u001B[0m \u001B[38;5;28;01mtry\u001B[39;00m:\n\u001B[1;32m 6\u001B[0m \u001B[38;5;66;03m# Get the last sample event\u001B[39;00m\n\u001B[0;32m----> 7\u001B[0m last_event_response \u001B[38;5;241m=\u001B[39m \u001B[43mapi_instance\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mget_last_sample_event_samples_samples_sample_id_events_last_get\u001B[49m(\u001B[38;5;241m14\u001B[39m)\n\u001B[1;32m 8\u001B[0m \u001B[38;5;28mprint\u001B[39m(\u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mThe response of get_last_sample_event:\u001B[39m\u001B[38;5;130;01m\\n\u001B[39;00m\u001B[38;5;124m\"\u001B[39m)\n\u001B[1;32m 9\u001B[0m pprint(last_event_response)\n",
|
|
"\u001B[0;31mAttributeError\u001B[0m: 'SamplesApi' object has no attribute 'get_last_sample_event_samples_samples_sample_id_events_last_get'"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 14
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-30T12:38:46.149389Z",
|
|
"start_time": "2025-01-30T12:38:46.110767Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": [
|
|
"from aareDBclient import ApiClient, SamplesApi # Import the appropriate client\n",
|
|
"from aareDBclient.rest import ApiException\n",
|
|
"import mimetypes\n",
|
|
"\n",
|
|
"# File path to the image\n",
|
|
"file_path = \"backend/tests/sample_image/IMG_1942.jpg\"\n",
|
|
"\n",
|
|
"# Sample ID\n",
|
|
"sample_id = 27 # Replace with a valid sample_id from your FastAPI backend\n",
|
|
"\n",
|
|
"# Initialize the API client\n",
|
|
"with ApiClient(configuration) as api_client:\n",
|
|
" api_instance = SamplesApi(api_client) # Adjust as per your API structure\n",
|
|
"\n",
|
|
" try:\n",
|
|
" # Open the file and read as binary\n",
|
|
" with open(file_path, \"rb\") as file:\n",
|
|
" # Get the MIME type for the file\n",
|
|
" mime_type, _ = mimetypes.guess_type(file_path)\n",
|
|
"\n",
|
|
" # Call the API method for uploading sample images\n",
|
|
" response = api_instance.upload_sample_images_samples_samples_sample_id_upload_images_post(\n",
|
|
" sample_id=sample_id,\n",
|
|
" uploaded_files=[file.read()] # Pass raw bytes as a list\n",
|
|
" )\n",
|
|
"\n",
|
|
" # Print the response from the API\n",
|
|
" print(\"API Response:\")\n",
|
|
" print(response)\n",
|
|
"\n",
|
|
" except ApiException as e:\n",
|
|
" # Handle API exception gracefully\n",
|
|
" print(\"Exception occurred while uploading the file:\")\n",
|
|
" print(f\"Status Code: {e.status}\")\n",
|
|
" if e.body:\n",
|
|
" print(f\"Error Details: {e.body}\")"
|
|
],
|
|
"id": "40404614d1a63f95",
|
|
"outputs": [
|
|
{
|
|
"ename": "ValueError",
|
|
"evalue": "Unsupported file value",
|
|
"output_type": "error",
|
|
"traceback": [
|
|
"\u001B[0;31m---------------------------------------------------------------------------\u001B[0m",
|
|
"\u001B[0;31mValueError\u001B[0m Traceback (most recent call last)",
|
|
"Cell \u001B[0;32mIn[19], line 22\u001B[0m\n\u001B[1;32m 19\u001B[0m mime_type, _ \u001B[38;5;241m=\u001B[39m mimetypes\u001B[38;5;241m.\u001B[39mguess_type(file_path)\n\u001B[1;32m 21\u001B[0m \u001B[38;5;66;03m# Call the API method for uploading sample images\u001B[39;00m\n\u001B[0;32m---> 22\u001B[0m response \u001B[38;5;241m=\u001B[39m \u001B[43mapi_instance\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mupload_sample_images_samples_samples_sample_id_upload_images_post\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 23\u001B[0m \u001B[43m \u001B[49m\u001B[43msample_id\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43msample_id\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 24\u001B[0m \u001B[43m \u001B[49m\u001B[43muploaded_files\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m[\u001B[49m\u001B[43mfile\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mread\u001B[49m\u001B[43m(\u001B[49m\u001B[43m)\u001B[49m\u001B[43m]\u001B[49m\u001B[43m \u001B[49m\u001B[38;5;66;43;03m# Pass raw bytes as a list\u001B[39;49;00m\n\u001B[1;32m 25\u001B[0m \u001B[43m\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 27\u001B[0m \u001B[38;5;66;03m# Print the response from the API\u001B[39;00m\n\u001B[1;32m 28\u001B[0m \u001B[38;5;28mprint\u001B[39m(\u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mAPI Response:\u001B[39m\u001B[38;5;124m\"\u001B[39m)\n",
|
|
"File \u001B[0;32m/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/pydantic/validate_call_decorator.py:60\u001B[0m, in \u001B[0;36mvalidate_call.<locals>.validate.<locals>.wrapper_function\u001B[0;34m(*args, **kwargs)\u001B[0m\n\u001B[1;32m 58\u001B[0m \u001B[38;5;129m@functools\u001B[39m\u001B[38;5;241m.\u001B[39mwraps(function)\n\u001B[1;32m 59\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21mwrapper_function\u001B[39m(\u001B[38;5;241m*\u001B[39margs, \u001B[38;5;241m*\u001B[39m\u001B[38;5;241m*\u001B[39mkwargs):\n\u001B[0;32m---> 60\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[43mvalidate_call_wrapper\u001B[49m\u001B[43m(\u001B[49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[43margs\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[38;5;241;43m*\u001B[39;49m\u001B[43mkwargs\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m/Library/Frameworks/Python.framework/Versions/3.12/lib/python3.12/site-packages/pydantic/_internal/_validate_call.py:96\u001B[0m, in \u001B[0;36mValidateCallWrapper.__call__\u001B[0;34m(self, *args, **kwargs)\u001B[0m\n\u001B[1;32m 95\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21m__call__\u001B[39m(\u001B[38;5;28mself\u001B[39m, \u001B[38;5;241m*\u001B[39margs: Any, \u001B[38;5;241m*\u001B[39m\u001B[38;5;241m*\u001B[39mkwargs: Any) \u001B[38;5;241m-\u001B[39m\u001B[38;5;241m>\u001B[39m Any:\n\u001B[0;32m---> 96\u001B[0m res \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m__pydantic_validator__\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mvalidate_python\u001B[49m\u001B[43m(\u001B[49m\u001B[43mpydantic_core\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mArgsKwargs\u001B[49m\u001B[43m(\u001B[49m\u001B[43margs\u001B[49m\u001B[43m,\u001B[49m\u001B[43m \u001B[49m\u001B[43mkwargs\u001B[49m\u001B[43m)\u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 97\u001B[0m \u001B[38;5;28;01mif\u001B[39;00m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39m__return_pydantic_validator__:\n\u001B[1;32m 98\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39m__return_pydantic_validator__(res)\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api/samples_api.py:874\u001B[0m, in \u001B[0;36mSamplesApi.upload_sample_images_samples_samples_sample_id_upload_images_post\u001B[0;34m(self, sample_id, uploaded_files, _request_timeout, _request_auth, _content_type, _headers, _host_index)\u001B[0m\n\u001B[1;32m 827\u001B[0m \u001B[38;5;129m@validate_call\u001B[39m\n\u001B[1;32m 828\u001B[0m \u001B[38;5;28;01mdef\u001B[39;00m\u001B[38;5;250m \u001B[39m\u001B[38;5;21mupload_sample_images_samples_samples_sample_id_upload_images_post\u001B[39m(\n\u001B[1;32m 829\u001B[0m \u001B[38;5;28mself\u001B[39m,\n\u001B[0;32m (...)\u001B[0m\n\u001B[1;32m 843\u001B[0m _host_index: Annotated[StrictInt, Field(ge\u001B[38;5;241m=\u001B[39m\u001B[38;5;241m0\u001B[39m, le\u001B[38;5;241m=\u001B[39m\u001B[38;5;241m0\u001B[39m)] \u001B[38;5;241m=\u001B[39m \u001B[38;5;241m0\u001B[39m,\n\u001B[1;32m 844\u001B[0m ) \u001B[38;5;241m-\u001B[39m\u001B[38;5;241m>\u001B[39m \u001B[38;5;28mobject\u001B[39m:\n\u001B[1;32m 845\u001B[0m \u001B[38;5;250m \u001B[39m\u001B[38;5;124;03m\"\"\"Upload Sample Images\u001B[39;00m\n\u001B[1;32m 846\u001B[0m \n\u001B[1;32m 847\u001B[0m \n\u001B[0;32m (...)\u001B[0m\n\u001B[1;32m 871\u001B[0m \u001B[38;5;124;03m :return: Returns the result object.\u001B[39;00m\n\u001B[1;32m 872\u001B[0m \u001B[38;5;124;03m \"\"\"\u001B[39;00m \u001B[38;5;66;03m# noqa: E501\u001B[39;00m\n\u001B[0;32m--> 874\u001B[0m _param \u001B[38;5;241m=\u001B[39m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43m_upload_sample_images_samples_samples_sample_id_upload_images_post_serialize\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 875\u001B[0m \u001B[43m \u001B[49m\u001B[43msample_id\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43msample_id\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 876\u001B[0m \u001B[43m \u001B[49m\u001B[43muploaded_files\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43muploaded_files\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 877\u001B[0m \u001B[43m \u001B[49m\u001B[43m_request_auth\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_request_auth\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 878\u001B[0m \u001B[43m \u001B[49m\u001B[43m_content_type\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_content_type\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 879\u001B[0m \u001B[43m \u001B[49m\u001B[43m_headers\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_headers\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 880\u001B[0m \u001B[43m \u001B[49m\u001B[43m_host_index\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_host_index\u001B[49m\n\u001B[1;32m 881\u001B[0m \u001B[43m \u001B[49m\u001B[43m)\u001B[49m\n\u001B[1;32m 883\u001B[0m _response_types_map: Dict[\u001B[38;5;28mstr\u001B[39m, Optional[\u001B[38;5;28mstr\u001B[39m]] \u001B[38;5;241m=\u001B[39m {\n\u001B[1;32m 884\u001B[0m \u001B[38;5;124m'\u001B[39m\u001B[38;5;124m200\u001B[39m\u001B[38;5;124m'\u001B[39m: \u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mobject\u001B[39m\u001B[38;5;124m\"\u001B[39m,\n\u001B[1;32m 885\u001B[0m \u001B[38;5;124m'\u001B[39m\u001B[38;5;124m422\u001B[39m\u001B[38;5;124m'\u001B[39m: \u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mHTTPValidationError\u001B[39m\u001B[38;5;124m\"\u001B[39m,\n\u001B[1;32m 886\u001B[0m }\n\u001B[1;32m 887\u001B[0m response_data \u001B[38;5;241m=\u001B[39m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39mapi_client\u001B[38;5;241m.\u001B[39mcall_api(\n\u001B[1;32m 888\u001B[0m \u001B[38;5;241m*\u001B[39m_param,\n\u001B[1;32m 889\u001B[0m _request_timeout\u001B[38;5;241m=\u001B[39m_request_timeout\n\u001B[1;32m 890\u001B[0m )\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api/samples_api.py:1096\u001B[0m, in \u001B[0;36mSamplesApi._upload_sample_images_samples_samples_sample_id_upload_images_post_serialize\u001B[0;34m(self, sample_id, uploaded_files, _request_auth, _content_type, _headers, _host_index)\u001B[0m\n\u001B[1;32m 1092\u001B[0m \u001B[38;5;66;03m# authentication setting\u001B[39;00m\n\u001B[1;32m 1093\u001B[0m _auth_settings: List[\u001B[38;5;28mstr\u001B[39m] \u001B[38;5;241m=\u001B[39m [\n\u001B[1;32m 1094\u001B[0m ]\n\u001B[0;32m-> 1096\u001B[0m \u001B[38;5;28;01mreturn\u001B[39;00m \u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mapi_client\u001B[49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mparam_serialize\u001B[49m\u001B[43m(\u001B[49m\n\u001B[1;32m 1097\u001B[0m \u001B[43m \u001B[49m\u001B[43mmethod\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[38;5;124;43m'\u001B[39;49m\u001B[38;5;124;43mPOST\u001B[39;49m\u001B[38;5;124;43m'\u001B[39;49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1098\u001B[0m \u001B[43m \u001B[49m\u001B[43mresource_path\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[38;5;124;43m'\u001B[39;49m\u001B[38;5;124;43m/samples/samples/\u001B[39;49m\u001B[38;5;132;43;01m{sample_id}\u001B[39;49;00m\u001B[38;5;124;43m/upload-images\u001B[39;49m\u001B[38;5;124;43m'\u001B[39;49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1099\u001B[0m \u001B[43m \u001B[49m\u001B[43mpath_params\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_path_params\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1100\u001B[0m \u001B[43m \u001B[49m\u001B[43mquery_params\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_query_params\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1101\u001B[0m \u001B[43m \u001B[49m\u001B[43mheader_params\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_header_params\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1102\u001B[0m \u001B[43m \u001B[49m\u001B[43mbody\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_body_params\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1103\u001B[0m \u001B[43m \u001B[49m\u001B[43mpost_params\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_form_params\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1104\u001B[0m \u001B[43m \u001B[49m\u001B[43mfiles\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_files\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1105\u001B[0m \u001B[43m \u001B[49m\u001B[43mauth_settings\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_auth_settings\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1106\u001B[0m \u001B[43m \u001B[49m\u001B[43mcollection_formats\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_collection_formats\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1107\u001B[0m \u001B[43m \u001B[49m\u001B[43m_host\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_host\u001B[49m\u001B[43m,\u001B[49m\n\u001B[1;32m 1108\u001B[0m \u001B[43m \u001B[49m\u001B[43m_request_auth\u001B[49m\u001B[38;5;241;43m=\u001B[39;49m\u001B[43m_request_auth\u001B[49m\n\u001B[1;32m 1109\u001B[0m \u001B[43m\u001B[49m\u001B[43m)\u001B[49m\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:214\u001B[0m, in \u001B[0;36mApiClient.param_serialize\u001B[0;34m(self, method, resource_path, path_params, query_params, header_params, body, post_params, files, auth_settings, collection_formats, _host, _request_auth)\u001B[0m\n\u001B[1;32m 209\u001B[0m post_params \u001B[38;5;241m=\u001B[39m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39mparameters_to_tuples(\n\u001B[1;32m 210\u001B[0m post_params,\n\u001B[1;32m 211\u001B[0m collection_formats\n\u001B[1;32m 212\u001B[0m )\n\u001B[1;32m 213\u001B[0m \u001B[38;5;28;01mif\u001B[39;00m files:\n\u001B[0;32m--> 214\u001B[0m post_params\u001B[38;5;241m.\u001B[39mextend(\u001B[38;5;28;43mself\u001B[39;49m\u001B[38;5;241;43m.\u001B[39;49m\u001B[43mfiles_parameters\u001B[49m\u001B[43m(\u001B[49m\u001B[43mfiles\u001B[49m\u001B[43m)\u001B[49m)\n\u001B[1;32m 216\u001B[0m \u001B[38;5;66;03m# auth setting\u001B[39;00m\n\u001B[1;32m 217\u001B[0m \u001B[38;5;28mself\u001B[39m\u001B[38;5;241m.\u001B[39mupdate_params_for_auth(\n\u001B[1;32m 218\u001B[0m header_params,\n\u001B[1;32m 219\u001B[0m query_params,\n\u001B[0;32m (...)\u001B[0m\n\u001B[1;32m 224\u001B[0m request_auth\u001B[38;5;241m=\u001B[39m_request_auth\n\u001B[1;32m 225\u001B[0m )\n",
|
|
"File \u001B[0;32m~/PycharmProjects/heidi-v2/backend/aareDBclient/api_client.py:554\u001B[0m, in \u001B[0;36mApiClient.files_parameters\u001B[0;34m(self, files)\u001B[0m\n\u001B[1;32m 552\u001B[0m filedata \u001B[38;5;241m=\u001B[39m v\n\u001B[1;32m 553\u001B[0m \u001B[38;5;28;01melse\u001B[39;00m:\n\u001B[0;32m--> 554\u001B[0m \u001B[38;5;28;01mraise\u001B[39;00m \u001B[38;5;167;01mValueError\u001B[39;00m(\u001B[38;5;124m\"\u001B[39m\u001B[38;5;124mUnsupported file value\u001B[39m\u001B[38;5;124m\"\u001B[39m)\n\u001B[1;32m 555\u001B[0m mimetype \u001B[38;5;241m=\u001B[39m (\n\u001B[1;32m 556\u001B[0m mimetypes\u001B[38;5;241m.\u001B[39mguess_type(filename)[\u001B[38;5;241m0\u001B[39m]\n\u001B[1;32m 557\u001B[0m \u001B[38;5;129;01mor\u001B[39;00m \u001B[38;5;124m'\u001B[39m\u001B[38;5;124mapplication/octet-stream\u001B[39m\u001B[38;5;124m'\u001B[39m\n\u001B[1;32m 558\u001B[0m )\n\u001B[1;32m 559\u001B[0m params\u001B[38;5;241m.\u001B[39mappend(\n\u001B[1;32m 560\u001B[0m \u001B[38;5;28mtuple\u001B[39m([k, \u001B[38;5;28mtuple\u001B[39m([filename, filedata, mimetype])])\n\u001B[1;32m 561\u001B[0m )\n",
|
|
"\u001B[0;31mValueError\u001B[0m: Unsupported file value"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 19
|
|
},
|
|
{
|
|
"metadata": {
|
|
"ExecuteTime": {
|
|
"end_time": "2025-01-20T15:14:51.219091Z",
|
|
"start_time": "2025-01-20T15:14:51.216755Z"
|
|
}
|
|
},
|
|
"cell_type": "code",
|
|
"source": "help(api_instance.upload_sample_images_samples_samples_sample_id_upload_images_post)",
|
|
"id": "8dd70634ffa5f37e",
|
|
"outputs": [
|
|
{
|
|
"name": "stdout",
|
|
"output_type": "stream",
|
|
"text": [
|
|
"Help on method upload_sample_images_samples_samples_sample_id_upload_images_post in module aareDBclient.api.samples_api:\n",
|
|
"\n",
|
|
"upload_sample_images_samples_samples_sample_id_upload_images_post(sample_id: Annotated[int, Strict(strict=True)], uploaded_files: List[Union[Annotated[bytes, Strict(strict=True)], Annotated[str, Strict(strict=True)]]], _request_timeout: Union[NoneType, Annotated[float, Strict(strict=True), FieldInfo(annotation=NoneType, required=True, metadata=[Gt(gt=0)])], Tuple[Annotated[float, Strict(strict=True), FieldInfo(annotation=NoneType, required=True, metadata=[Gt(gt=0)])], Annotated[float, Strict(strict=True), FieldInfo(annotation=NoneType, required=True, metadata=[Gt(gt=0)])]]] = None, _request_auth: Optional[Dict[Annotated[str, Strict(strict=True)], Any]] = None, _content_type: Optional[Annotated[str, Strict(strict=True)]] = None, _headers: Optional[Dict[Annotated[str, Strict(strict=True)], Any]] = None, _host_index: Annotated[int, Strict(strict=True), FieldInfo(annotation=NoneType, required=True, metadata=[Ge(ge=0), Le(le=0)])] = 0) -> object method of aareDBclient.api.samples_api.SamplesApi instance\n",
|
|
" Upload Sample Images\n",
|
|
"\n",
|
|
" Uploads images for a sample and stores them in a directory structure: images/user/date/dewar_name/puck_name/position/. Args: sample_id (int): ID of the sample. uploaded_files (Union[List[UploadFile], List[bytes]]): List of image files (as UploadFile or raw bytes). db (Session): SQLAlchemy database session.\n",
|
|
"\n",
|
|
" :param sample_id: (required)\n",
|
|
" :type sample_id: int\n",
|
|
" :param uploaded_files: (required)\n",
|
|
" :type uploaded_files: List[bytearray]\n",
|
|
" :param _request_timeout: timeout setting for this request. If one\n",
|
|
" number provided, it will be total request\n",
|
|
" timeout. It can also be a pair (tuple) of\n",
|
|
" (connection, read) timeouts.\n",
|
|
" :type _request_timeout: int, tuple(int, int), optional\n",
|
|
" :param _request_auth: set to override the auth_settings for an a single\n",
|
|
" request; this effectively ignores the\n",
|
|
" authentication in the spec for a single request.\n",
|
|
" :type _request_auth: dict, optional\n",
|
|
" :param _content_type: force content-type for the request.\n",
|
|
" :type _content_type: str, Optional\n",
|
|
" :param _headers: set to override the headers for a single\n",
|
|
" request; this effectively ignores the headers\n",
|
|
" in the spec for a single request.\n",
|
|
" :type _headers: dict, optional\n",
|
|
" :param _host_index: set to override the host_index for a single\n",
|
|
" request; this effectively ignores the host_index\n",
|
|
" in the spec for a single request.\n",
|
|
" :type _host_index: int, optional\n",
|
|
" :return: Returns the result object.\n",
|
|
"\n"
|
|
]
|
|
}
|
|
],
|
|
"execution_count": 51
|
|
}
|
|
],
|
|
"metadata": {
|
|
"kernelspec": {
|
|
"display_name": "Python 3",
|
|
"language": "python",
|
|
"name": "python3"
|
|
},
|
|
"language_info": {
|
|
"codemirror_mode": {
|
|
"name": "ipython",
|
|
"version": 2
|
|
},
|
|
"file_extension": ".py",
|
|
"mimetype": "text/x-python",
|
|
"name": "python",
|
|
"nbconvert_exporter": "python",
|
|
"pygments_lexer": "ipython2",
|
|
"version": "2.7.6"
|
|
}
|
|
},
|
|
"nbformat": 4,
|
|
"nbformat_minor": 5
|
|
}
|