mirror of
https://github.com/slsdetectorgroup/slsDetectorPackage.git
synced 2025-04-22 14:38:14 +02:00
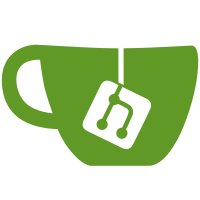
git-svn-id: file:///afs/psi.ch/project/sls_det_software/svn/slsDetectorSoftware@6 951219d9-93cf-4727-9268-0efd64621fa3
167 lines
4.5 KiB
C++
167 lines
4.5 KiB
C++
|
|
#ifndef EIGER_DETECTOR_H
|
|
#define EIGER_DETECTOR_H
|
|
|
|
using namespace std;
|
|
|
|
/**
|
|
*
|
|
* @libdoc The eigerDetector class contains Eiger specific functions.
|
|
*
|
|
* @short This is class contains all Eiger specific functions
|
|
* @author Ian Johnson
|
|
* @version 0.1
|
|
*/
|
|
|
|
|
|
|
|
#include "slsDetector.h"
|
|
|
|
class eigerDetector : public slsDetector{
|
|
|
|
|
|
|
|
public:
|
|
|
|
|
|
|
|
/** (default) constructor
|
|
|
|
\param id is the detector index which is needed to define the shared memory id. Different physical detectors should have different IDs in order to work independently
|
|
|
|
|
|
*/
|
|
eigerDetector(int id=0);
|
|
//slsDetector(string const fname);
|
|
/** destructor */
|
|
~eigerDetector(){};
|
|
|
|
|
|
|
|
/**
|
|
reads a trim file
|
|
\param fname name of the file to be read
|
|
\param myMod pointer to the module structure which has to be set. <BR> If it is NULL a new module structure will be created
|
|
\returns the pointer to myMod or NULL if reading the file failed
|
|
*/
|
|
|
|
sls_detector_module* readTrimFile(string fname, sls_detector_module* myMod=NULL);
|
|
|
|
/**
|
|
writes a trim file
|
|
\param fname name of the file to be written
|
|
\param mod module structure which has to be written to file
|
|
\returns OK or FAIL if the file could not be written
|
|
|
|
\sa ::sls_detector_module
|
|
*/
|
|
int writeTrimFile(string fname, sls_detector_module mod);
|
|
|
|
/**
|
|
writes a trim file for module number imod - the values will be read from the current detector structure
|
|
\param fname name of the file to be written
|
|
\param imod module number
|
|
\returns OK or FAIL if the file could not be written
|
|
\sa ::sls_detector_module sharedSlsDetector
|
|
*/
|
|
int writeTrimFile(string fname, int imod);
|
|
|
|
|
|
/**
|
|
writes a data file
|
|
\param name of the file to be written
|
|
\param data array of data values
|
|
\param err array of arrors on the data. If NULL no errors will be written
|
|
|
|
\param ang array of angular values. If NULL data will be in the form chan-val(-err) otherwise ang-val(-err)
|
|
\param dataformat format of the data: can be 'i' integer or 'f' float (default)
|
|
\param nch number of channels to be written to file. if -1 defaults to the number of installed channels of the detector
|
|
\returns OK or FAIL if it could not write the file or data=NULL
|
|
|
|
|
|
*/
|
|
int writeDataFile(string fname, float *data, float *err=NULL, float *ang=NULL, char dataformat='f', int nch=-1);
|
|
|
|
/**
|
|
writes a data file
|
|
\param name of the file to be written
|
|
\param data array of data values
|
|
\returns OK or FAIL if it could not write the file or data=NULL
|
|
*/
|
|
int writeDataFile(string fname, int *data);
|
|
|
|
/**
|
|
reads a data file
|
|
\param name of the file to be read
|
|
\param data array of data values to be filled
|
|
\param err array of arrors on the data. If NULL no errors are expected on the file
|
|
|
|
\param ang array of angular values. If NULL data are expected in the form chan-val(-err) otherwise ang-val(-err)
|
|
\param dataformat format of the data: can be 'i' integer or 'f' float (default)
|
|
\param nch number of channels to be written to file. if <=0 defaults to the number of installed channels of the detector
|
|
\returns OK or FAIL if it could not read the file or data=NULL
|
|
|
|
|
|
*/
|
|
int readDataFile(string fname, float *data, float *err=NULL, float *ang=NULL, char dataformat='f', int nch=0);
|
|
|
|
/**
|
|
reads a data file
|
|
\param name of the file to be read
|
|
\param data array of data values
|
|
\returns OK or FAIL if it could not read the file or data=NULL
|
|
*/
|
|
int readDataFile(string fname, int *data);
|
|
|
|
/**
|
|
returns the location of the calibration files
|
|
\sa sharedSlsDetector
|
|
*/
|
|
|
|
/**
|
|
int readCalibrationFile(string fname, float &gain, float &offset);
|
|
|
|
|
|
\param fname file to be read
|
|
\param gain reference to the gain variable
|
|
\offset reference to the offset variable
|
|
\sa sharedSlsDetector
|
|
*/
|
|
int readCalibrationFile(string fname, float &gain, float &offset);
|
|
|
|
|
|
/**
|
|
writes a clibration file
|
|
\param fname file to be written
|
|
\param gain
|
|
\param offset
|
|
\sa sharedSlsDetector
|
|
*/
|
|
int writeCalibrationFile(string fname, float gain, float offset);
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
/**
|
|
decode data from the detector converting them to an array of floats, one for each channle
|
|
\param datain data from the detector
|
|
\returns pointer to a float array with a data per channel
|
|
*/
|
|
float* decodeData(int *datain);
|
|
|
|
|
|
|
|
|
|
|
|
private:
|
|
|
|
|
|
};
|
|
|
|
|
|
#endif
|