mirror of
https://github.com/slsdetectorgroup/slsDetectorPackage.git
synced 2025-04-28 09:10:01 +02:00
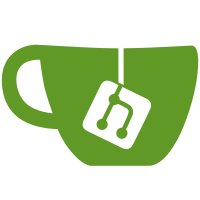
* fix for editingFinished for qlineedit using isModified() * spinbox disable keyboard tracking to use valuechanged so slot called only after editing finished, focus fix * return pressed forces qtextfield to be set (slot for tab checks for modification flag due to avoid unnecessary set when focus). This is to remove inconsistencies from command line. A return should set even if it looks like no modification in gui Co-authored-by: Erik Fröjdh <erik.frojdh@gmail.com>
95 lines
2.6 KiB
C++
95 lines
2.6 KiB
C++
#include "qDacWidget.h"
|
|
#include "qDefs.h"
|
|
|
|
qDacWidget::qDacWidget(QWidget *parent, sls::Detector *detector, bool d,
|
|
std::string n, slsDetectorDefs::dacIndex i)
|
|
: QWidget(parent), det(detector), isDac(d), index(i) {
|
|
setupUi(this);
|
|
SetupWidgetWindow(n);
|
|
}
|
|
|
|
qDacWidget::~qDacWidget() {}
|
|
|
|
void qDacWidget::SetupWidgetWindow(std::string name) {
|
|
lblDac->setText(name.c_str());
|
|
if (isDac) {
|
|
spinDac->setDecimals(0);
|
|
} else {
|
|
spinDac->setSuffix(0x00b0 + QString("C"));
|
|
spinDac->setReadOnly(true);
|
|
lblDacmV->setMinimumWidth(0);
|
|
lblDacmV->setMaximumWidth(0);
|
|
}
|
|
Initialization();
|
|
Refresh();
|
|
}
|
|
|
|
void qDacWidget::Initialization() {
|
|
if (isDac) {
|
|
connect(spinDac, SIGNAL(valueChanged(double)), this, SLOT(SetDac()));
|
|
}
|
|
}
|
|
|
|
void qDacWidget::SetDetectorIndex(int id) {
|
|
detectorIndex = id;
|
|
Refresh();
|
|
}
|
|
|
|
void qDacWidget::GetDac() {
|
|
LOG(logDEBUG) << "Getting Dac " << index;
|
|
|
|
disconnect(spinDac, SIGNAL(valueChanged(double)), this, SLOT(SetDac()));
|
|
try {
|
|
// dac units
|
|
auto retval = det->getDAC(index, 0, {detectorIndex}).squash(-1);
|
|
spinDac->setValue(retval);
|
|
// mv
|
|
retval = det->getDAC(index, 1, {detectorIndex}).squash(-1);
|
|
// -6 is the minimum amt of space it occupies, if more needed, its
|
|
// padded with ' ', negative value for left aligned text
|
|
lblDacmV->setText(QString("%1mV").arg(retval, -6));
|
|
}
|
|
CATCH_DISPLAY(std::string("Could not get dac ") + std::to_string(index),
|
|
"qDacWidget::GetDac")
|
|
|
|
connect(spinDac, SIGNAL(valueChanged(double)), this, SLOT(SetDac()));
|
|
}
|
|
|
|
void qDacWidget::SetDac() {
|
|
int val = (int)spinDac->value();
|
|
LOG(logINFO) << "Setting dac:" << lblDac->text().toAscii().data() << " : "
|
|
<< val;
|
|
|
|
try {
|
|
det->setDAC(index, val, 0, {detectorIndex});
|
|
}
|
|
CATCH_DISPLAY(std::string("Could not set dac ") + std::to_string(index),
|
|
"qDacWidget::SetDac")
|
|
|
|
// update mV anyway
|
|
GetDac();
|
|
}
|
|
|
|
void qDacWidget::GetAdc() {
|
|
LOG(logDEBUG) << "Getting ADC " << index;
|
|
|
|
try {
|
|
auto retval = det->getTemperature(index, {detectorIndex}).squash(-1);
|
|
if (retval == -1 && detectorIndex == -1) {
|
|
spinDac->setValue(-1);
|
|
} else {
|
|
spinDac->setValue(retval);
|
|
}
|
|
}
|
|
CATCH_DISPLAY(std::string("Could not get adc ") + std::to_string(index),
|
|
"qDacWidget::GetAdc")
|
|
}
|
|
|
|
void qDacWidget::Refresh() {
|
|
if (isDac) {
|
|
GetDac();
|
|
} else {
|
|
GetAdc();
|
|
}
|
|
}
|