mirror of
https://github.com/slsdetectorgroup/slsDetectorPackage.git
synced 2025-07-13 11:21:50 +02:00
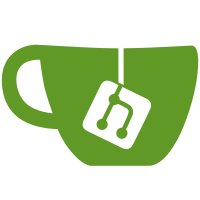
* badchannels for m3 and modify for g2 (file from single and multi) * m3: invert polarity of bit 7 and 11 signals from setmodule, allow commas in bad channel file * badchannel file can take commas, colons and comments (also taking care of spaces at the end of channel numbers) * tests 'badchannels' and 'Channel file reading' added, removing duplicates in badchannel list, defining macro for num counters in client side * fix segfault when list from file is empty, * fix tests assertion for ctbconfig (adding message) for c++11 * fixed badchannels in m3server (clocking in trimming) * badchannel tests can be run from any folder (finds the file)
63 lines
1.4 KiB
C++
63 lines
1.4 KiB
C++
#include "catch.hpp"
|
|
#include <string>
|
|
|
|
#include <stdlib.h>
|
|
|
|
#include "CtbConfig.h"
|
|
#include "SharedMemory.h"
|
|
#include <fstream>
|
|
|
|
namespace sls {
|
|
|
|
TEST_CASE("Default construction") {
|
|
static_assert(sizeof(CtbConfig) == 360,
|
|
"Size of CtbConfig does not match"); // 18*20
|
|
|
|
CtbConfig c;
|
|
auto names = c.getDacNames();
|
|
REQUIRE(names.size() == 18);
|
|
REQUIRE(names[0] == "dac0");
|
|
REQUIRE(names[1] == "dac1");
|
|
REQUIRE(names[2] == "dac2");
|
|
REQUIRE(names[3] == "dac3");
|
|
}
|
|
|
|
TEST_CASE("Set and get a single dac name") {
|
|
CtbConfig c;
|
|
c.setDacName(3, "vrf");
|
|
auto names = c.getDacNames();
|
|
|
|
REQUIRE(c.getDacName(3) == "vrf");
|
|
REQUIRE(names[3] == "vrf");
|
|
}
|
|
|
|
TEST_CASE("Set a name that is too large throws") {
|
|
CtbConfig c;
|
|
REQUIRE_THROWS(c.setDacName(3, "somestringthatisreallytolongforadatac"));
|
|
}
|
|
|
|
TEST_CASE("Length of dac name cannot be 0") {
|
|
CtbConfig c;
|
|
REQUIRE_THROWS(c.setDacName(1, ""));
|
|
}
|
|
|
|
TEST_CASE("Copy a CTB config") {
|
|
CtbConfig c1;
|
|
c1.setDacName(5, "somename");
|
|
|
|
auto c2 = c1;
|
|
// change the name on the first object
|
|
// to detecto shallow copy
|
|
c1.setDacName(5, "someothername");
|
|
REQUIRE(c2.getDacName(5) == "somename");
|
|
}
|
|
|
|
TEST_CASE("Move CtbConfig ") {
|
|
CtbConfig c1;
|
|
c1.setDacName(3, "yetanothername");
|
|
CtbConfig c2(std::move(c1));
|
|
REQUIRE(c2.getDacName(3) == "yetanothername");
|
|
}
|
|
|
|
} // namespace sls
|