mirror of
https://github.com/slsdetectorgroup/slsDetectorPackage.git
synced 2025-04-22 14:38:14 +02:00
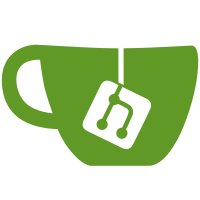
* badchannels for m3 and modify for g2 (file from single and multi) * m3: invert polarity of bit 7 and 11 signals from setmodule, allow commas in bad channel file * badchannel file can take commas, colons and comments (also taking care of spaces at the end of channel numbers) * tests 'badchannels' and 'Channel file reading' added, removing duplicates in badchannel list, defining macro for num counters in client side * fix segfault when list from file is empty, * fix tests assertion for ctbconfig (adding message) for c++11 * fixed badchannels in m3server (clocking in trimming) * badchannel tests can be run from any folder (finds the file)
44 lines
1.1 KiB
C++
44 lines
1.1 KiB
C++
// SPDX-License-Identifier: LGPL-3.0-or-other
|
|
// Copyright (C) 2021 Contributors to the SLS Detector Package
|
|
#include "catch.hpp"
|
|
#include "sls/file_utils.h"
|
|
#include <iostream>
|
|
#include <stdio.h>
|
|
#include <unistd.h>
|
|
#include <vector>
|
|
|
|
#include "tests/globals.h"
|
|
|
|
namespace sls {
|
|
|
|
TEST_CASE("Get size of empty file") {
|
|
char fname[] = "temfile_XXXXXX";
|
|
std::ifstream ifs(fname);
|
|
auto size = getFileSize(ifs);
|
|
REQUIRE(size <= 0); // -1 or zero
|
|
}
|
|
|
|
TEST_CASE("Get size of file with data") {
|
|
constexpr size_t n_bytes = 137;
|
|
std::vector<char> data(n_bytes);
|
|
char fname[] = "temfile_XXXXXX";
|
|
int fh = mkstemp(fname);
|
|
write(fh, data.data(), n_bytes);
|
|
|
|
std::ifstream ifs(fname);
|
|
auto size = getFileSize(ifs);
|
|
REQUIRE(size == n_bytes);
|
|
REQUIRE(ifs.tellg() == 0); // getting size resets pos!
|
|
}
|
|
|
|
TEST_CASE("Channel file reading") {
|
|
std::string fname =
|
|
getAbsolutePathFromCurrentProcess(TEST_FILE_NAME_BAD_CHANNELS);
|
|
std::vector<int> list;
|
|
REQUIRE_NOTHROW(list = getChannelsFromFile(fname));
|
|
std::vector<int> expected = {0, 12, 15, 40, 41, 42, 43, 44, 1279};
|
|
REQUIRE(list == expected);
|
|
}
|
|
|
|
} // namespace sls
|