mirror of
https://github.com/slsdetectorgroup/slsDetectorPackage.git
synced 2025-04-23 15:00:02 +02:00
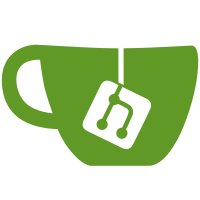
* WIP * wip * wip * removed loop * sending 1 frame * send multiple frames * c11 for server * WIP * WIP * Add CMake for the simulators. Requires some refactoring to make slsDetectorServer a proper library. * Working cmake for jungfrau * Working cmake for eiger * WIP * WIP * Add preliminary working eiger simulator and breaks the jungfrau simulator * virtual servers connected * changes to make it work for rhel7 and install binaries in bin * removed some unnecessary prints * removed binaries and virtual makefiles
144 lines
3.3 KiB
C
Executable File
144 lines
3.3 KiB
C
Executable File
/* A simple server in the internet domain using TCP
|
|
The port number is passed as an argument */
|
|
|
|
#include "sls_detector_defs.h"
|
|
#include "logger.h"
|
|
#include "communication_funcs.h"
|
|
#include "slsDetectorServer_funcs.h"
|
|
#include "slsDetectorServer_defs.h"
|
|
#include "versionAPI.h"
|
|
|
|
#include <signal.h>
|
|
#include <string.h>
|
|
|
|
|
|
// Global variables from communication_funcs
|
|
extern int isControlServer;
|
|
extern int ret;
|
|
|
|
// Global variables from slsDetectorServer_funcs
|
|
extern int sockfd;
|
|
extern int debugflag;
|
|
|
|
// Global variables from slsDetectorFunctionList
|
|
#ifdef GOTTHARDD
|
|
extern int phaseShift;
|
|
#endif
|
|
|
|
void error(char *msg){
|
|
perror(msg);
|
|
}
|
|
|
|
int main(int argc, char *argv[]){
|
|
// print version
|
|
if (argc > 1 && !strcasecmp(argv[1], "-version")) {
|
|
int version = 0;
|
|
#ifdef GOTTHARDD
|
|
version = APIGOTTHARD;
|
|
#elif EIGERD
|
|
version = APIEIGER;
|
|
#elif JUNGFRAUD
|
|
version = APIJUNGFRAU;
|
|
#elif CHIPTESTBOARDD
|
|
version = APICTB;
|
|
#elif MOENCHD
|
|
version = APIMOENCH;
|
|
#endif
|
|
FILE_LOG(logINFO, ("SLS Detector Server %s (0x%x)\n", GITBRANCH, version));
|
|
}
|
|
|
|
int portno = DEFAULT_PORTNO;
|
|
int retval = OK;
|
|
int fd = 0;
|
|
|
|
// if socket crash, ignores SISPIPE, prevents global signal handler
|
|
// subsequent read/write to socket gives error - must handle locally
|
|
signal(SIGPIPE, SIG_IGN);
|
|
|
|
// circumvent the basic tests
|
|
{
|
|
int i;
|
|
for (i = 1; i < argc; ++i) {
|
|
if(!strcasecmp(argv[i],"-stopserver")) {
|
|
FILE_LOG(logINFO, ("Detected stop server\n"));
|
|
isControlServer = 0;
|
|
}
|
|
else if(!strcasecmp(argv[i],"-devel")){
|
|
FILE_LOG(logINFO, ("Detected developer mode\n"));
|
|
debugflag = 1;
|
|
}
|
|
#ifdef GOTTHARDD
|
|
else if(!strcasecmp(argv[i],"-phaseshift")){
|
|
if ((i + 1) >= argc) {
|
|
FILE_LOG(logERROR, ("no phase shift value given. Exiting.\n"));
|
|
return -1;
|
|
}
|
|
if (sscanf(argv[i + 1], "%d", &phaseShift) == 0) {
|
|
FILE_LOG(logERROR, ("cannot decode phase shift value %s. Exiting.\n", argv[i + 1]));
|
|
return -1;
|
|
}
|
|
FILE_LOG(logINFO, ("Detected phase shift of %d\n", phaseShift));
|
|
}
|
|
#endif
|
|
}
|
|
}
|
|
|
|
#ifdef STOP_SERVER
|
|
char cmd[100];
|
|
memset(cmd, 0, 100);
|
|
#endif
|
|
if (isControlServer) {
|
|
portno = DEFAULT_PORTNO;
|
|
FILE_LOG(logINFO, ("Opening control server on port %d \n", portno));
|
|
#ifdef STOP_SERVER
|
|
{
|
|
int i;
|
|
for (i = 0; i < argc; ++i)
|
|
sprintf(cmd, "%s %s", cmd, argv[i]);
|
|
sprintf(cmd,"%s -stopserver&", cmd);
|
|
FILE_LOG(logDEBUG1, ("Command to start stop server:%s\n", cmd));
|
|
system(cmd);
|
|
}
|
|
#endif
|
|
} else {
|
|
portno = DEFAULT_PORTNO + 1;
|
|
FILE_LOG(logINFO,("Opening stop server on port %d \n", portno));
|
|
}
|
|
|
|
init_detector();
|
|
|
|
{ // bind socket
|
|
sockfd = bindSocket(portno);
|
|
if (ret == FAIL)
|
|
return -1;
|
|
}
|
|
|
|
// assign function table
|
|
function_table();
|
|
|
|
if (isControlServer) {
|
|
FILE_LOG(logINFOBLUE, ("Control Server Ready...\n\n"));
|
|
} else {
|
|
FILE_LOG(logINFO, ("Stop Server Ready...\n\n"));
|
|
}
|
|
|
|
// waits for connection
|
|
while(retval != GOODBYE && retval != REBOOT) {
|
|
fd = acceptConnection(sockfd);
|
|
if (fd > 0) {
|
|
retval = decode_function(fd);
|
|
closeConnection(fd);
|
|
}
|
|
}
|
|
|
|
exitServer(sockfd);
|
|
|
|
if (retval == REBOOT) {
|
|
FILE_LOG(logINFOBLUE,("Rebooting!\n"));
|
|
fflush(stdout);
|
|
system("reboot");
|
|
}
|
|
FILE_LOG(logINFO,("Goodbye!\n"));
|
|
return 0;
|
|
}
|