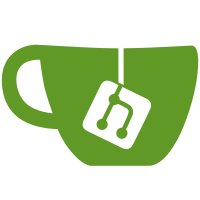
The dhcp server is systemd-networkd, and the dhcp plugin can request an ip but can not renew it. The systemd-networkd just ignore the renew request. ``` 2024/09/14 21:46:00 no DHCP packet received within 10s 2024/09/14 21:46:00 retrying in 31.529038 seconds 2024/09/14 21:46:42 no DHCP packet received within 10s 2024/09/14 21:46:42 retrying in 63.150490 seconds 2024/09/14 21:47:45 98184616c91f15419f5cacd012697f85afaa2daeb5d3233e28b0ec21589fb45a/iot/eth1: no more tries 2024/09/14 21:47:45 98184616c91f15419f5cacd012697f85afaa2daeb5d3233e28b0ec21589fb45a/iot/eth1: renewal time expired, rebinding 2024/09/14 21:47:45 Link "eth1" down. Attempting to set up 2024/09/14 21:47:45 98184616c91f15419f5cacd012697f85afaa2daeb5d3233e28b0ec21589fb45a/iot/eth1: lease rebound, expiration is 2024-09-14 22:47:45.309270751 +0800 CST m=+11730.048516519 ``` Follow the https://datatracker.ietf.org/doc/html/rfc2131#section-4.3.6, following options must not be sent in renew - Requested IP Address - Server Identifier Since the upstream code has been inactive for 6 years, we should switch to another dhcpv4 library. The new selected one is https://github.com/insomniacslk/dhcp. Signed-off-by: Songmin Li <lisongmin@protonmail.com>
78 lines
2.6 KiB
Go
78 lines
2.6 KiB
Go
package iana
|
|
|
|
// StatusCode represents a IANA status code for DHCPv6
|
|
//
|
|
// IANA Status Codes for DHCPv6
|
|
// https://www.iana.org/assignments/dhcpv6-parameters/dhcpv6-parameters.xhtml#dhcpv6-parameters-5
|
|
type StatusCode uint16
|
|
|
|
// IANA status codes
|
|
const (
|
|
// RFC 3315 par. 24..4
|
|
StatusSuccess StatusCode = 0
|
|
StatusUnspecFail StatusCode = 1
|
|
StatusNoAddrsAvail StatusCode = 2
|
|
StatusNoBinding StatusCode = 3
|
|
StatusNotOnLink StatusCode = 4
|
|
StatusUseMulticast StatusCode = 5
|
|
StatusNoPrefixAvail StatusCode = 6
|
|
// RFC 5007
|
|
StatusUnknownQueryType StatusCode = 7
|
|
StatusMalformedQuery StatusCode = 8
|
|
StatusNotConfigured StatusCode = 9
|
|
StatusNotAllowed StatusCode = 10
|
|
// RFC 5460
|
|
StatusQueryTerminated StatusCode = 11
|
|
// RFC 7653
|
|
StatusDataMissing StatusCode = 12
|
|
StatusCatchUpComplete StatusCode = 13
|
|
StatusNotSupported StatusCode = 14
|
|
StatusTLSConnectionRefused StatusCode = 15
|
|
// RFC 8156
|
|
StatusAddressInUse StatusCode = 16
|
|
StatusConfigurationConflict StatusCode = 17
|
|
StatusMissingBindingInformation StatusCode = 18
|
|
StatusOutdatedBindingInformation StatusCode = 19
|
|
StatusServerShuttingDown StatusCode = 20
|
|
StatusDNSUpdateNotSupported StatusCode = 21
|
|
StatusExcessiveTimeSkew StatusCode = 22
|
|
)
|
|
|
|
// String returns a mnemonic name for a given status code
|
|
func (s StatusCode) String() string {
|
|
if sc := statusCodeToStringMap[s]; sc != "" {
|
|
return sc
|
|
}
|
|
return "Unknown"
|
|
}
|
|
|
|
var statusCodeToStringMap = map[StatusCode]string{
|
|
StatusSuccess: "Success",
|
|
StatusUnspecFail: "UnspecFail",
|
|
StatusNoAddrsAvail: "NoAddrsAvail",
|
|
StatusNoBinding: "NoBinding",
|
|
StatusNotOnLink: "NotOnLink",
|
|
StatusUseMulticast: "UseMulticast",
|
|
StatusNoPrefixAvail: "NoPrefixAvail",
|
|
// RFC 5007
|
|
StatusUnknownQueryType: "UnknownQueryType",
|
|
StatusMalformedQuery: "MalformedQuery",
|
|
StatusNotConfigured: "NotConfigured",
|
|
StatusNotAllowed: "NotAllowed",
|
|
// RFC 5460
|
|
StatusQueryTerminated: "QueryTerminated",
|
|
// RFC 7653
|
|
StatusDataMissing: "DataMissing",
|
|
StatusCatchUpComplete: "CatchUpComplete",
|
|
StatusNotSupported: "NotSupported",
|
|
StatusTLSConnectionRefused: "TLSConnectionRefused",
|
|
// RFC 8156
|
|
StatusAddressInUse: "AddressInUse",
|
|
StatusConfigurationConflict: "ConfigurationConflict",
|
|
StatusMissingBindingInformation: "MissingBindingInformation",
|
|
StatusOutdatedBindingInformation: "OutdatedBindingInformation",
|
|
StatusServerShuttingDown: "ServerShuttingDown",
|
|
StatusDNSUpdateNotSupported: "DNSUpdateNotSupported",
|
|
StatusExcessiveTimeSkew: "ExcessiveTimeSkew",
|
|
}
|